stm32f407使用I2C2驱动PCA9685产生16通道PWM的代码
时间: 2023-12-10 17:05:27 浏览: 202
以下是使用STM32F407的I2C2驱动PCA9685产生16通道PWM的代码示例:
```c
#include "stm32f4xx.h"
#define PCA9685_ADDRESS 0x80 // PCA9685 I2C address
// PCA9685 Registers
#define MODE1 0x00
#define PRESCALE 0xFE
#define LED0_ON_L 0x06
I2C_HandleTypeDef hi2c2;
void PCA9685_Init(void)
{
uint8_t data[2];
// Set the MODE1 register to put the PCA9685 in sleep mode
data[0] = MODE1;
data[1] = 0x10; // Sleep mode
HAL_I2C_Master_Transmit(&hi2c2, PCA9685_ADDRESS, data, 2, 100);
// Set the prescaler to generate a 50Hz PWM frequency
data[0] = PRESCALE;
data[1] = 0x79; // Prescaler value for 50Hz
HAL_I2C_Master_Transmit(&hi2c2, PCA9685_ADDRESS, data, 2, 100);
// Set the MODE1 register to restart the PCA9685
data[0] = MODE1;
data[1] = 0x00; // Restart
HAL_I2C_Master_Transmit(&hi2c2, PCA9685_ADDRESS, data, 2, 100);
}
void PCA9685_SetPWM(uint8_t channel, uint16_t on_time, uint16_t off_time)
{
uint8_t data[5];
// Set the ON time for the specified channel
data[0] = LED0_ON_L + 4 * channel;
data[1] = on_time & 0xFF;
data[2] = on_time >> 8;
HAL_I2C_Master_Transmit(&hi2c2, PCA9685_ADDRESS, data, 3, 100);
// Set the OFF time for the specified channel
data[0] = LED0_ON_L + 4 * channel + 2;
data[1] = off_time & 0xFF;
data[2] = off_time >> 8;
HAL_I2C_Master_Transmit(&hi2c2, PCA9685_ADDRESS, data, 3, 100);
}
int main(void)
{
// Initialize the I2C2 peripheral
hi2c2.Instance = I2C2;
hi2c2.Init.ClockSpeed = 400000;
hi2c2.Init.DutyCycle = I2C_DUTYCYCLE_2;
hi2c2.Init.OwnAddress1 = 0;
hi2c2.Init.AddressingMode = I2C_ADDRESSINGMODE_7BIT;
hi2c2.Init.DualAddressMode = I2C_DUALADDRESS_DISABLE;
hi2c2.Init.OwnAddress2 = 0;
hi2c2.Init.GeneralCallMode = I2C_GENERALCALL_DISABLE;
hi2c2.Init.NoStretchMode = I2C_NOSTRETCH_DISABLE;
HAL_I2C_Init(&hi2c2);
// Initialize the PCA9685
PCA9685_Init();
// Set the PWM values for channels 0-15
PCA9685_SetPWM(0, 0, 4095); // Channel 0 full on
PCA9685_SetPWM(1, 0, 2047); // Channel 1 half on
PCA9685_SetPWM(2, 2047, 0); // Channel 2 half off
// ...
while (1)
{
// Main loop
}
}
```
在此示例中,我们使用STM32F407的I2C2外设来驱动PCA9685芯片,以产生16个通道的PWM信号。首先,我们需要定义PCA9685芯片的I2C地址以及其寄存器地址。然后,我们定义了两个函数,PCA9685_Init()和PCA9685_SetPWM(),用于初始化PCA9685芯片并设置PWM输出。在主函数中,我们首先初始化I2C2外设,然后调用PCA9685_Init()函数以初始化PCA9685芯片。最后,我们调用PCA9685_SetPWM()函数来设置16个PWM通道的输出。
请注意,此示例中使用的PWM频率为50Hz,可以根据需要进行更改。此外,我们只设置了前三个PWM通道的输出,您可以根据需要设置其他通道的输出。
阅读全文
相关推荐
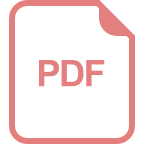
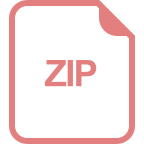





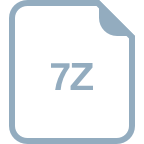
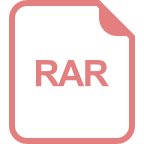
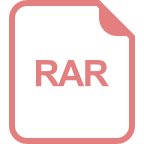
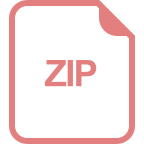
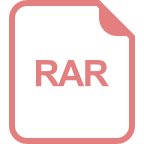
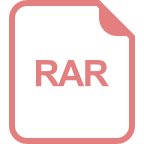
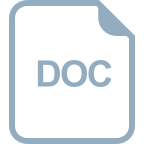
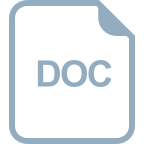

