请使用二叉树的先序遍历算法,统计二叉树中叶子节点的个数(假设二叉树已经使用链式储存,根节点的指针为Btree*root,不需要函数模板。函数原型:void leaf_count(Btree*root,int&k)。其中指针root指向二叉树的根结点,参数k为统计叶子结点数
时间: 2023-12-03 18:44:13 浏览: 75
好的,下面是使用二叉树的先序遍历算法统计叶子节点个数的代码:
```c++
struct Btree {
int data;
Btree *left;
Btree *right;
};
void leaf_count(Btree *root, int &k) {
if (root == nullptr) {
return;
}
if (root->left == nullptr && root->right == nullptr) {
k++;
}
leaf_count(root->left, k);
leaf_count(root->right, k);
}
```
其中,函数递归地访问二叉树的每一个结点,并判断该结点是否为叶子结点。如果是叶子结点,就让计数器k加1。最终,k的值即为二叉树中叶子结点的个数。
相关问题
请使用二叉树的先序遍历算法,统计二叉树中叶子节点的个数(假设二叉树已经使用链式储存,根节点的指针为Btree*root,不需要函数模板。
以下是使用递归的二叉树先序遍历算法,统计二叉树中叶子节点的个数:
```cpp
int countLeaves(Btree* root) {
if (root == nullptr) {
return 0;
}
if (root->left == nullptr && root->right == nullptr) {
return 1;
}
return countLeaves(root->left) + countLeaves(root->right);
}
```
其中,Btree 是二叉树节点的结构体,包含指向左右子树的指针。该算法通过递归实现,如果当前节点为叶子节点,则返回 1,否则递归计算左右子树中叶子节点的个数,并相加返回。最终得到的结果即为二叉树中叶子节点的个数。
请使用二叉树的先序遍历算法,统计二叉树中叶子节点的个数(假设二叉树已经使用链式存储,根节点的指针为Btree * root)。不需要函数模板。函数原型:void leaf_count(Btree *root,int & k)其中指针root指向二叉树的根节点,参数k为统计叶子节点数?
下面是使用先序遍历算法统计二叉树中叶子节点数的函数leaf_count的实现:
```C++
struct Btree {
int val;
Btree *left;
Btree *right;
};
void leaf_count(Btree *root, int &k) {
if(root == nullptr) {
return;
}
if(root->left == nullptr && root->right == nullptr) {
k++;
}
leaf_count(root->left, k);
leaf_count(root->right, k);
}
```
首先判断当前节点是否为叶子节点,如果是,则将叶子节点数k加1。然后对左子树和右子树递归调用leaf_count函数,统计左右子树中的叶子节点数。最终,叶子节点数即为参数k的值。
阅读全文
相关推荐
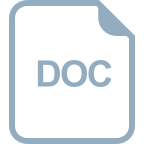
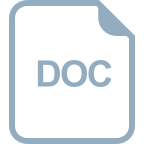
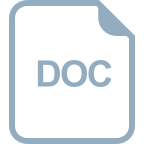


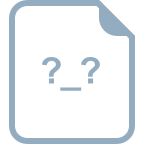
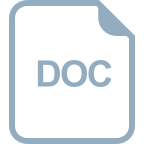
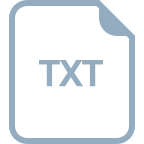
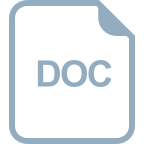
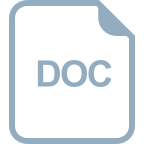
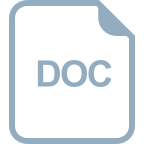





