在opencv中使用c++对提取出的轮廓用外接矩形框选,矩形框命名为火龙果,计算出矩形中心点坐标,返回矩形中心点坐标值
时间: 2023-05-31 14:06:24 浏览: 138
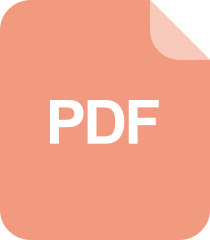
opencv3/C++轮廓的提取与筛选方式

以下是使用C++和OpenCV实现提取轮廓并框选的示例代码:
```c++
#include <opencv2/opencv.hpp>
#include <iostream>
using namespace cv;
using namespace std;
int main()
{
Mat image = imread("test.jpg"); // 读取图像
if (image.empty()) // 判断图像是否读取成功
{
cout << "Failed to read image" << endl;
return -1;
}
Mat gray;
cvtColor(image, gray, COLOR_BGR2GRAY); // 转换为灰度图像
Mat thresholded;
threshold(gray, thresholded, 0, 255, THRESH_BINARY | THRESH_OTSU); // 二值化
Mat canny;
Canny(thresholded, canny, 100, 200); // 边缘检测
vector<vector<Point>> contours;
findContours(canny, contours, RETR_EXTERNAL, CHAIN_APPROX_SIMPLE); // 提取轮廓
Mat result = image.clone();
for (size_t i = 0; i < contours.size(); i++)
{
Rect rect = boundingRect(contours[i]); // 计算外接矩形
rectangle(result, rect, Scalar(0, 255, 0), 2); // 绘制矩形
putText(result, "Fire Dragon Fruit", Point(rect.x, rect.y - 5), FONT_HERSHEY_SIMPLEX, 0.5, Scalar(0, 255, 0), 2); // 标注矩形名字
Point center(rect.x + rect.width / 2, rect.y + rect.height / 2); // 计算矩形中心点坐标
circle(result, center, 2, Scalar(0, 0, 255), 2); // 绘制中心点
cout << "Center point of Fire Dragon Fruit " << i + 1 << ": (" << center.x << ", " << center.y << ")" << endl; // 输出中心点坐标
}
imshow("Result", result); // 显示结果
waitKey(0);
return 0;
}
```
其中,`boundingRect`函数用于计算外接矩形,`rectangle`函数用于绘制矩形,`putText`函数用于标注矩形名字,`circle`函数用于绘制中心点。计算矩形中心点坐标的代码为:
```c++
Point center(rect.x + rect.width / 2, rect.y + rect.height / 2);
```
其中,`rect.x`和`rect.y`分别是矩形左上角的坐标,`rect.width`和`rect.height`分别是矩形的宽度和高度。
阅读全文
相关推荐

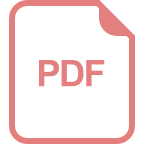
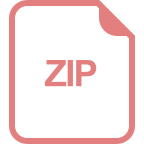
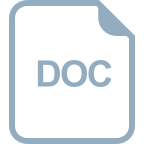









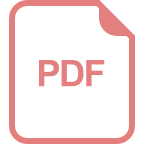

