请帮我用python语言A*算法实现一个八数码问题
时间: 2023-06-02 11:03:10 浏览: 101
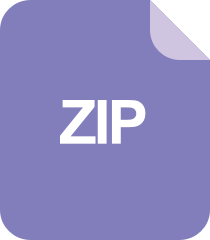
基于Python实现的AStar求解八数码问题.zip

以下是一个八数码问题的A*算法实现:
```
import heapq
# 八数码问题的状态类
class State:
def __init__(self, board, moves, heuristic):
self.board = board
self.moves = moves
self.heuristic = heuristic
self.priority = len(moves) + heuristic
# 定义比较运算符,用于堆排序
def __lt__(self, other):
return self.priority < other.priority
# 计算当前状态的启发式值
def heuristic(board):
return sum(abs(i // 3 - board[i] // 3) + abs(i % 3 - board[i] % 3) for i in range(9) if board[i])
# A*算法求解八数码问题
def solve(initial_board):
heap = []
visited = set()
heapq.heappush(heap, State(initial_board, [], heuristic(initial_board)))
while heap:
state = heapq.heappop(heap)
if state.board == [0, 1, 2, 3, 4, 5, 6, 7, 8]:
return state.moves
if str(state.board) in visited:
continue
visited.add(str(state.board))
i = state.board.index(0)
if i % 3 > 0:
new_board = state.board[:]
new_board[i], new_board[i-1] = new_board[i-1], new_board[i]
heapq.heappush(heap, State(new_board, state.moves + ['L'], heuristic(new_board)))
if i % 3 < 2:
new_board = state.board[:]
new_board[i], new_board[i+1] = new_board[i+1], new_board[i]
heapq.heappush(heap, State(new_board, state.moves + ['R'], heuristic(new_board)))
if i // 3 > 0:
new_board = state.board[:]
new_board[i], new_board[i-3] = new_board[i-3], new_board[i]
heapq.heappush(heap, State(new_board, state.moves + ['U'], heuristic(new_board)))
if i // 3 < 2:
new_board = state.board[:]
new_board[i], new_board[i+3] = new_board[i+3], new_board[i]
heapq.heappush(heap, State(new_board, state.moves + ['D'], heuristic(new_board)))
return None
# 测试
initial_board = [1, 2, 3, 4, 5, 6, 0, 7, 8]
moves = solve(initial_board)
if moves:
print('解: ' + '->'.join(moves))
else:
print('无解')
```
在上面的实现中,我们使用了一个`State`类来表示八数码问题的状态,其中包括当前的棋盘状态、移动序列、启发式值和优先级。我们使用一个堆来保存待扩展的状态,每次从堆中选取优先级最高的状态进行扩展。在扩展过程中,我们首先判断当前状态是否为目标状态,如果是则返回移动序列;否则将当前状态标记为已访问,并生成所有可能的后继状态,将它们加入堆中等待扩展。在生成后继状态时,我们将0所在位置向上、下、左、右四个方向移动,并更新移动序列和启发式值,然后将新状态加入堆中。在计算启发式值时,我们使用了曼哈顿距离作为评价函数,即将每个数字移动到目标位置所需的最小步数之和。
阅读全文
相关推荐
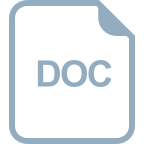
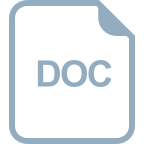

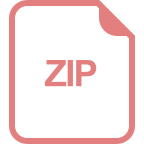
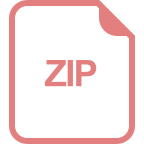
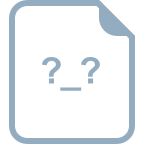
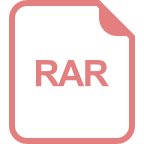







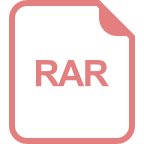
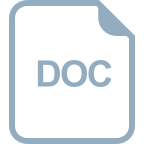
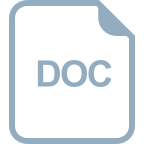
