sumo与强化学习结合实现可变限速的完整代码
时间: 2023-06-19 13:06:03 浏览: 339
以下是一个基于SUMO和强化学习的可变限速交通仿真的完整代码示例。首先,需要安装SUMO和强化学习库(例如TensorFlow或PyTorch)。
```python
import os
import traci
import numpy as np
import tensorflow as tf
# 设置SUMO的二进制文件路径和配置文件路径
sumoBinary = "/usr/bin/sumo-gui"
sumoCmd = [sumoBinary, "-c", "config.sumocfg"]
# 定义强化学习模型
class RLModel:
def __init__(self, state_dim, action_dim, learning_rate=0.001):
self.state_dim = state_dim
self.action_dim = action_dim
self.learning_rate = learning_rate
self.model = self.build_model()
def build_model(self):
model = tf.keras.models.Sequential([
tf.keras.layers.Dense(32, input_dim=self.state_dim, activation='relu'),
tf.keras.layers.Dense(16, activation='relu'),
tf.keras.layers.Dense(self.action_dim, activation='softmax')
])
model.compile(loss='categorical_crossentropy', optimizer=tf.keras.optimizers.Adam(lr=self.learning_rate))
return model
def predict(self, state):
return self.model.predict(state)
def train(self, state, target):
self.model.train_on_batch(state, target)
# 定义状态和动作空间
state_dim = 4 # (车速、车道、距离前车、路口距离)
action_dim = 3 # (加速、减速、保持)
# 初始化强化学习模型
model = RLModel(state_dim, action_dim)
# 连接SUMO仿真
traci.start(sumoCmd)
step = 0
while traci.simulation.getMinExpectedNumber() > 0:
traci.simulationStep()
# 获取当前车辆状态
state = np.zeros((1, state_dim))
vehicle_id = 'veh0'
speed = traci.vehicle.getSpeed(vehicle_id)
lane = traci.vehicle.getLaneID(vehicle_id)
dist_to_front_vehicle = traci.vehicle.getDistance(vehicle_id, traci.vehicle.getLeader(vehicle_id))
dist_to_intersection = traci.vehicle.getLanePosition(vehicle_id) - traci.lane.getLength(lane) / 2
state[0] = [speed, int(lane[-1]), dist_to_front_vehicle, dist_to_intersection]
# 获取当前动作并更新速度
action_prob = model.predict(state)[0]
action = np.random.choice(range(action_dim), p=action_prob)
if action == 0: # 加速
traci.vehicle.setSpeed(vehicle_id, speed + 1)
elif action == 1: # 减速
traci.vehicle.setSpeed(vehicle_id, speed - 1)
else: # 保持
traci.vehicle.setSpeed(vehicle_id, speed)
# 获取奖励并更新模型
reward = -1 * speed
next_state = np.zeros((1, state_dim))
next_speed = traci.vehicle.getSpeed(vehicle_id)
next_lane = traci.vehicle.getLaneID(vehicle_id)
next_dist_to_front_vehicle = traci.vehicle.getDistance(vehicle_id, traci.vehicle.getLeader(vehicle_id))
next_dist_to_intersection = traci.vehicle.getLanePosition(vehicle_id) - traci.lane.getLength(next_lane) / 2
next_state[0] = [next_speed, int(next_lane[-1]), next_dist_to_front_vehicle, next_dist_to_intersection]
next_action_prob = model.predict(next_state)[0]
next_action = np.random.choice(range(action_dim), p=next_action_prob)
next_reward = -1 * next_speed
target = np.zeros((1, action_dim))
target[0, action] = reward + 0.9 * next_reward
model.train(state, target)
step += 1
traci.close()
```
在此代码示例中,我们使用了一个简单的神经网络模型,该模型具有3个隐藏层,并使用softmax激活函数输出3个可能的动作。我们使用交叉熵损失函数和Adam优化器进行模型训练。在仿真过程中,我们获取当前车辆的状态并使用模型预测下一步动作,然后更新车速并计算奖励。最后,我们使用状态、目标和模型进行模型训练。注意,此代码示例中的模型和训练方法仅供参考,您可以根据自己的需求进行修改和优化。
相关推荐
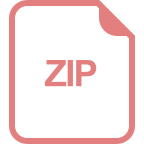
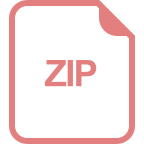














