编写求二叉树高度的函数
时间: 2023-09-25 14:12:58 浏览: 140
好的,以下是求二叉树高度的函数的Python代码实现:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
def get_height(root):
if not root:
return 0
left_height = get_height(root.left)
right_height = get_height(root.right)
return max(left_height, right_height) + 1
```
其中,`TreeNode`为二叉树节点的类定义,`get_height`为求二叉树高度的函数,`root`为根节点。函数的实现采用递归的方式,分别计算左子树和右子树的高度,取两者中的较大值并加上1即为整棵树的高度。如果根节点为空,则返回0。
相关问题
用c语言1.编写函数,输入字符序列,建立二叉树的二叉链表 2.编写函数,实现二叉树的中序递归遍历算法。 3.编写函数,实现二叉树的中序非递归遍历算法 4.编写函数,借助队列实现二叉树的层次遍历算法 5.编写函数,求二叉树的高度 6.编写函数,求二叉树的结点个数 7.编写函数,求二叉树的叶子个数 8.编写函数,交换二叉树每个结点的左子树和右子树
这些问题属于编程类问题,我可以回答。
1. 下面是用C语言编写输入字符序列,建立二叉树的二叉链表的函数:
```c
typedef struct BiNode {
char data;
struct BiNode *left;
struct BiNode *right;
} BiNode, *BiTree;
void createBiTree(BiTree *root) {
char ch;
scanf("%c", &ch);
if (ch == '#') {
*root = NULL;
} else {
*root = (BiNode *) malloc(sizeof(BiNode));
(*root)->data = ch;
createBiTree(&((*root)->left));
createBiTree(&((*root)->right));
}
}
```
2. 下面是用C语言编写实现二叉树的中序递归遍历算法的函数:
```c
void inOrder(BiTree tree) {
if (tree != NULL) {
inOrder(tree->left);
printf("%c ", tree->data);
inOrder(tree->right);
}
}
```
3. 下面是用C语言编写实现二叉树的中序非递归遍历算法的函数:
```c
void inOrderNonRecursion(BiTree tree) {
BiNode *stack[10000];
int top = -1;
BiNode *p = tree;
while (p != NULL || top != -1) {
while (p != NULL) {
stack[++top] = p;
p = p->left;
}
if (top != -1) {
p = stack[top--];
printf("%c ", p->data);
p = p->right;
}
}
}
```
4. 下面是用C语言编写借助队列实现二叉树的层次遍历算法的函数:
```c
void levelOrder(BiTree tree) {
BiNode *queue[10000];
int front = 0, rear = 0;
if (tree != NULL) {
queue[++rear] = tree;
while (front != rear) {
front++;
BiNode *p = queue[front];
printf("%c ", p->data);
if (p->left != NULL) {
queue[++rear] = p->left;
}
if (p->right != NULL) {
queue[++rear] = p->right;
}
}
}
}
```
5. 下面是用C语言编写求二叉树的高度的函数:
```c
int getHeight(BiTree tree) {
if (tree == NULL) {
return 0;
} else {
int leftHeight = getHeight(tree->left);
int rightHeight = getHeight(tree->right);
return leftHeight > rightHeight ? leftHeight + 1 : rightHeight + 1;
}
}
```
6. 下面是用C语言编写求二叉树的结点个数的函数:
```c
int getNodeCount(BiTree tree) {
if (tree == NULL) {
return 0;
} else {
return getNodeCount(tree->left) + getNodeCount(tree->right) + 1;
}
}
```
7. 下面是用C语言编写求二叉树的叶子个数的函数:
```c
int getLeafCount(BiTree tree) {
if (tree == NULL) {
return 0;
}
if (tree->left == NULL && tree->right == NULL) {
return 1;
} else {
return getLeafCount(tree->left) + getLeafCount(tree->right);
}
}
```
8. 下面是用C语言编写交换二叉树每个结点的左子树和右子树的函数:
```c
void swapBiTree(BiTree tree) {
if (tree != NULL) {
BiNode *temp = tree->left;
tree->left = tree->right;
tree->right = temp;
swapBiTree(tree->left);
swapBiTree(tree->right);
}
}
```
请用c语言编写一个求二叉树高度的算法
好的,以下是用C语言编写一个求二叉树高度的算法:
```C
#include <stdio.h>
#include <stdlib.h>
// 结构体定义
typedef struct Node {
int data;
struct Node* left;
struct Node* right;
} Node;
// 创建二叉树
Node* createTree() {
int data;
Node* root = NULL;
scanf("%d", &data);
if (data != -1) {
root = (Node*) malloc(sizeof(Node));
root->data = data;
root->left = createTree();
root->right = createTree();
}
return root;
}
// 递归求二叉树高度
int getHeight(Node* root) {
if (root == NULL) {
return 0;
} else {
int leftHeight = getHeight(root->left);
int rightHeight = getHeight(root->right);
return (leftHeight > rightHeight) ? (leftHeight + 1) : (rightHeight + 1);
}
}
// 主函数
int main() {
Node* root = createTree();
printf("Height of tree is %d\n", getHeight(root));
return 0;
}
```
以上代码中,createTree函数可以通过输入数组递归创建一个二叉树,并返回根节点。getHeight函数用递归的方法计算整个二叉树的高度,其中参数root表示根节点。最后,我们在主函数中调用createTree函数和getHeight函数,并输出结果。
阅读全文
相关推荐
















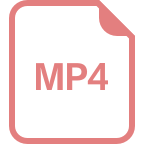