C语言编写求给定二叉树的高度
时间: 2024-12-24 18:33:07 浏览: 2
在C语言中,计算给定二叉树的高度通常采用递归的方法。二叉树的高度是指从根节点到最远叶子节点的最长路径上的边数。下面是基本的步骤:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义二叉树结构体
typedef struct TreeNode {
int val; // 节点值
struct TreeNode* left; // 左子节点指针
struct TreeNode* right; // 右子节点指针
} TreeNode;
// 计算树高度的辅助函数
int height(TreeNode* root) {
// 基本情况:空树的高度为0
if (root == NULL)
return 0;
// 通过递归分别计算左子树和右子树的高度,并取最大值加一作为当前节点的高度
int left_height = height(root->left);
int right_height = height(root->right);
// 返回两部分中较大者加上1(根节点)
return (left_height > right_height ? left_height : right_height) + 1;
}
// 主函数示例
int main() {
// 创建并初始化二叉树...
// (这里省略了创建二叉树的具体操作)
TreeNode* root = ...; // 树的根节点
int treeHeight = height(root);
printf("The height of the binary tree is %d.\n", treeHeight);
return 0;
}
```
阅读全文
相关推荐
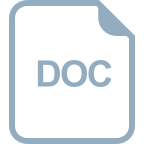
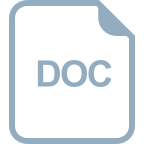
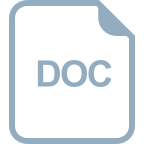















