5、给出一个使用JDK动态代理的例子。 6、(可选)给出使用CGlib动态代理的例子 注意事项: (1)请在程序中加入必要的注释和说明; (2)请采用规范一致的编程风格编写代码。
时间: 2024-02-12 22:05:34 浏览: 26
5、JDK动态代理示例:
```
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
// 定义一个接口
interface Hello {
void sayHello();
}
// 实现Hello接口的类
class HelloImpl implements Hello {
public void sayHello() {
System.out.println("Hello World!");
}
}
// 实现InvocationHandler接口的代理类
class HelloProxy implements InvocationHandler {
private Object target;
public HelloProxy(Object target) {
this.target = target;
}
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
System.out.println("Before invoke method: " + method.getName());
Object result = method.invoke(target, args);
System.out.println("After invoke method: " + method.getName());
return result;
}
}
public class Main {
public static void main(String[] args) {
// 创建实现Hello接口的类实例
Hello hello = new HelloImpl();
// 创建InvocationHandler实例
InvocationHandler handler = new HelloProxy(hello);
// 创建代理类实例
Hello proxy = (Hello) Proxy.newProxyInstance(
hello.getClass().getClassLoader(),
hello.getClass().getInterfaces(),
handler);
// 调用代理类的方法
proxy.sayHello();
}
}
```
6、CGlib动态代理示例:
```
import net.sf.cglib.proxy.Enhancer;
import net.sf.cglib.proxy.MethodInterceptor;
import net.sf.cglib.proxy.MethodProxy;
import java.lang.reflect.Method;
// 实现Hello接口的类
class Hello {
public void sayHello() {
System.out.println("Hello World!");
}
}
// 实现MethodInterceptor接口的代理类
class HelloProxy implements MethodInterceptor {
public Object intercept(Object obj, Method method, Object[] args, MethodProxy proxy) throws Throwable {
System.out.println("Before invoke method: " + method.getName());
Object result = proxy.invokeSuper(obj, args);
System.out.println("After invoke method: " + method.getName());
return result;
}
}
public class Main {
public static void main(String[] args) {
// 创建Enhancer实例
Enhancer enhancer = new Enhancer();
enhancer.setSuperclass(Hello.class);
enhancer.setCallback(new HelloProxy());
// 创建代理类实例
Hello proxy = (Hello) enhancer.create();
// 调用代理类的方法
proxy.sayHello();
}
}
```
相关推荐
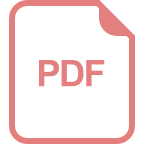














