用C++编写一个六子棋行棋的估值函数
时间: 2024-06-09 22:10:40 浏览: 169
六子棋的估值函数一般采用基于博弈树搜索的Minimax算法结合Alpha-Beta剪枝算法进行优化。在这里,我们简单介绍一下六子棋的估值函数实现过程。
首先,我们需要定义一个叫做board的二维数组来表示棋盘状态。其中,0表示空位,1表示黑子,2表示白子。接下来,我们可以定义一个估值函数来评估当前棋盘状态的好坏程度。
估值函数的实现主要是通过对当前棋盘状态进行分析,计算出当前状态下双方的胜率。常用的估值方法有两种:一种是基于棋型的估值方法,另一种是基于搜索的估值方法。这里我们介绍第一种方法。
首先,我们需要定义一些棋型,例如“眠三”、“活三”、“眠四”、“活四”等等。然后,我们可以遍历整个棋盘,统计黑子和白子在每一个方向上的棋型数目,然后计算出每个棋型的权重,最后加权求和得到估值函数的值。
下面是一个简单的估值函数的代码实现:
```c
int evaluate(int board[BOARD_SIZE][BOARD_SIZE], int player)
{
int score = 0;
int count1, count2, empty;
for (int i = 0; i < BOARD_SIZE; i++) {
for (int j = 0; j < BOARD_SIZE; j++) {
if (board[i][j] == player) {
// 统计横向棋型
count1 = countChess(board, i, j, 1, 0, player);
count2 = countChess(board, i, j, -1, 0, player);
score += evaluateChess(count1 + count2);
// 统计纵向棋型
count1 = countChess(board, i, j, 0, 1, player);
count2 = countChess(board, i, j, 0, -1, player);
score += evaluateChess(count1 + count2);
// 统计正斜向棋型
count1 = countChess(board, i, j, 1, 1, player);
count2 = countChess(board, i, j, -1, -1, player);
score += evaluateChess(count1 + count2);
// 统计反斜向棋型
count1 = countChess(board, i, j, -1, 1, player);
count2 = countChess(board, i, j, 1, -1, player);
score += evaluateChess(count1 + count2);
}
}
}
return score;
}
int evaluateChess(int count)
{
if (count >= 5) {
return 100000;
} else if (count == 4) {
return 10000;
} else if (count == 3) {
return 1000;
} else if (count == 2) {
return 100;
} else if (count == 1) {
return 10;
} else {
return 1;
}
}
int countChess(int board[BOARD_SIZE][BOARD_SIZE], int x, int y, int dx, int dy, int player)
{
int count = 0;
int empty = 0;
while (x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE && board[x][y] == player) {
x += dx;
y += dy;
count++;
}
if (x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE && board[x][y] == 0) {
empty++;
}
x -= dx;
y -= dy;
while (x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE && board[x][y] == player) {
x -= dx;
y -= dy;
count++;
}
if (x >= 0 && x < BOARD_SIZE && y >= 0 && y < BOARD_SIZE && board[x][y] == 0) {
empty++;
}
return count;
}
```
其中,countChess函数用于统计某个位置上某个方向上的棋型数目;evaluateChess函数用于计算某个棋型的权重;evaluate函数用于遍历整个棋盘,统计出每个棋型的数目并加权求和得到估值函数的值。
当然,这只是一个简单的估值函数,具体的实现还需要根据实际情况进行调整和优化。
阅读全文
相关推荐






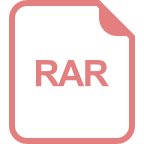
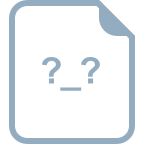
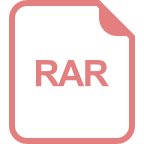






