用c语言写一段代码,函数名为void sort_Chars;形式参数为Chars arr [], Uint arrLen, int (* compChars)(const char *, Uint, const char *, Uint);Sort an array of Chars according to a function of comparing two Chars. ; After the computation, the elements of arr are sorted, from small to large, according to compChars.
时间: 2023-02-16 14:08:23 浏览: 123
你好!下面是一段使用 C 语言实现的代码,它实现了一个名为 sort_Chars 的函数,用于对数组 arr 中的字符进行排序。形式参数 arrLen 为数组 arr 的长度,compChars 为一个比较两个字符的函数。在计算完成后,根据 compChars 函数的比较结果,arr 中的元素按照从小到大的顺序进行排序。
```
#include <stdio.h>
#include <stdlib.h>
typedef unsigned int Uint;
typedef char Chars;
/* 比较两个字符的函数,若 a 小于 b,返回 -1;若 a 等于 b,返回 0;若 a 大于 b,返回 1 */
int compChars(const char *a, Uint aLen, const char *b, Uint bLen) {
if (*a < *b) {
return -1;
} else if (*a > *b) {
return 1;
} else {
return 0;
}
}
/* 对数组 arr 中的字符进行排序 */
void sort_Chars(Chars arr[], Uint arrLen, int (*compChars)(const char *, Uint, const char *, Uint)) {
int i, j;
Chars temp;
for (i = 0; i < arrLen - 1; i++) {
for (j = 0; j < arrLen - 1 - i; j++) {
if (compChars(&arr[j], 1, &arr[j + 1], 1) > 0) {
temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
}
int main() {
Chars chars[] = {'c', 'b', 'a', 'd', 'e'};
Uint charsLen = sizeof(chars) / sizeof(Chars);
sort_Chars(chars, charsLen, compChars);
for (int i = 0; i < charsLen; i++) {
printf("%c ", chars[i]);
}
printf("\n");
return 0;
}
```
相关推荐
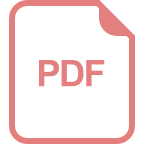
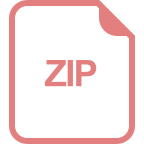
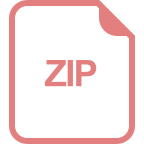














