//fl.8.4.cpp #include<iostream> #include<cstring> //为了使用strlen()、strcpy() using namespace std; struct stringy { char* str; //指向字符串 int ct; //字符串的长度(不包括‘\0’) }; void set(stringy&, char*); void show(const string&, int n = 0); void show(const stringy&, int n = 0); //set()、show()、show()的原型 int main() { stringy beany; char testing[] = "Reality isn't what it used to be."; set(beany, testing); //引用的第一个参数 //分配空间来保存testing的副本, //使用beany的str成员指向新的块, //把testing复制到新的块,并设置beany的ct成员 show(beany); //指向成员字符串1次 show(beany, 2); //指向成员字符串2次 testing[0] = 'D'; testing[1] = 'u'; show(testing); //指向testing字符串1次 show(testing,3); //指向testing字符串3次 show("Done!"); //beany内的创建的动态内存未回收,可在程序结束前使用delete回收 //例如:delete beany.str; return 0; } void show(const string& st, int n) { if (n == 0)n++; for (int i = 0; i < n; i++) { cout << st << endl; } } //输出string类型对象的信息 void show(const stringy& sty, int n) { if (n == 0)n++; for (int i = 0; i < n; i++) { cout << sty.str << endl; } } void set(stringy& sty, char* st) { sty.ct = strlen(st); sty.str = new char[sty.ct]; //通过new创建动态储存,此处不考虑回收 strcpy(sty.str, st); //复制字符串内容 }指出其中的错误并改正
时间: 2024-01-07 10:02:22 浏览: 23
在第24行和第28行的show函数中,应该将参数类型改为const stringy&而不是const string&,因为我们要输出的是stringy结构体中的字符串而不是一个string对象。
修改后的代码如下:
```cpp
//fl.8.4.cpp
#include<iostream>
#include<cstring> //为了使用strlen()、strcpy()
using namespace std;
struct stringy {
char* str; //指向字符串
int ct; //字符串的长度(不包括‘\0’)
};
void set(stringy&, char*);
void show(const string&, int n = 0);
void show(const stringy&, int n = 0); //set()、show()、show()的原型
int main() {
stringy beany;
char testing[] = "Reality isn't what it used to be.";
set(beany, testing); //引用的第一个参数
//分配空间来保存testing的副本,
//使用beany的str成员指向新的块,
//把testing复制到新的块,并设置beany的ct成员
show(beany); //指向成员字符串1次
show(beany, 2); //指向成员字符串2次
testing[0] = 'D';
testing[1] = 'u';
show(testing); //指向testing字符串1次
show(testing,3); //指向testing字符串3次
show("Done!");
//beany内的创建的动态内存未回收,可在程序结束前使用delete回收
//例如:delete beany.str;
delete [] beany.str; //释放动态内存
return 0;
}
void show(const stringy& sty, int n) {
if (n == 0) n++;
for (int i = 0; i < n; i++) {
cout << sty.str << endl;
}
}
void set(stringy& sty, char* st) {
sty.ct = strlen(st);
sty.str = new char[sty.ct + 1]; //申请的空间要多1,用来保存'\0'
//通过new创建动态储存,此处不考虑回收
strcpy(sty.str, st); //复制字符串内容
}
void show(const string& st, int n) {
if (n == 0) n++;
for (int i = 0; i < n; i++) {
cout << st << endl;
}
}
```
相关推荐
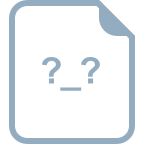















