当多个不同的图片的轮廓提取出来后,怎样对这些多个轮廓进行平均化或者拟合化的操作,最终输出一个最佳的轮廓图,请用python代码实现,并给出示例和解释
时间: 2024-05-24 17:13:43 浏览: 112
以下是ChitGPT的回答:
可以使用OpenCV库中的函数cv2.findContours()来提取所有图片的轮廓,并将它们存储在一个列表中。接下来,可以使用cv2.approxPolyDP()函数来对每个轮廓进行多边形拟合,从而得到一个近似的轮廓。最后,可以使用cv2.drawContours()函数将所有轮廓绘制在一张空白图像上,从而得到平均化或者拟合化后的轮廓图。以下是一个示例代码:
import cv2
# 读取所有图片
img1 = cv2.imread('image1.jpg')
img2 = cv2.imread('image2.jpg')
img3 = cv2.imread('image3.jpg')
# 提取所有图片的轮廓
contours1, _ = cv2.findContours(img1, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours2, _ = cv2.findContours(img2, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours3, _ = cv2.findContours(img3, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
# 对每个轮廓进行多边形拟合
approx_contours1 = cv2.approxPolyDP(contours1[0], 0.01*cv2.arcLength(contours1[0], True), True)
approx_contours2 = cv2.approxPolyDP(contours2[0], 0.01*cv2.arcLength(contours2[0], True), True)
approx_contours3 = cv2.approxPolyDP(contours3[0], 0.01*cv2.arcLength(contours3[0], True), True)
# 将所有轮廓绘制在一张空白图像上
height, width, _ = img1.shape
result = np.zeros((height, width, 3), np.uint8)
cv2.drawContours(result, [approx_contours1], -1, (255, 0, 0), 2)
cv2.drawContours(result, [approx_contours2], -1, (0, 255, 0), 2)
cv2.drawContours(result, [approx_contours3], -1, (0, 0, 255), 2)
# 显示结果
cv2.imshow('result', result)
cv2.waitKey(0)
cv2.destroyAllWindows()
这段代码将读取三张名为image1.jpg、image2.jpg和image3.jpg的图片,并将它们的轮廓提取出来。然后,对每个轮廓进行多边形拟合,并将它们绘制在一张空白图像上。最终的结果将显示在一个窗口中。
阅读全文
相关推荐
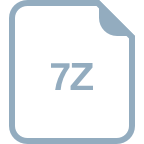
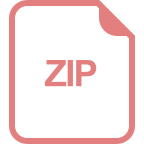
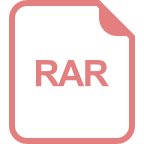
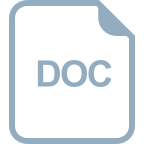
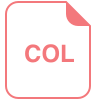
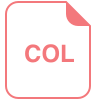
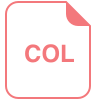
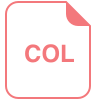
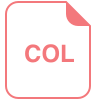
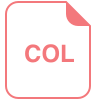
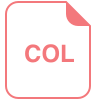
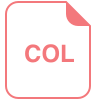
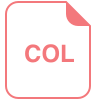
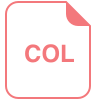
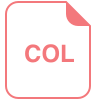
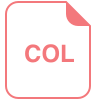
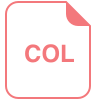