ARCore应用,给定一张图片作为标记,扫描生成预制体的一个实例。给出详细步骤,和代码。代码要有注释
时间: 2024-03-01 21:53:46 浏览: 18
步骤如下:
1. 首先,需要使用Unity创建一个ARCore项目,并导入Google提供的ARCore插件。
2. 创建一个空的场景,并在场景中添加一个ARCore Device Prefab(ARCore设备预制体)。
3. 创建一个Image Anchor(图像锚点),并将其设置为检测用的图像。
4. 创建一个预制体,并将其设置为Image Anchor的子物体。
5. 编写脚本,将预制体添加到Image Anchor上。
6. 在ARCore Device Prefab上添加AR Session和AR Session Origin组件,并将Image Anchor添加到AR Session Origin的子物体中。
7. 扫描标记图像,生成预制体的一个实例。
以下是代码示例:
```csharp
using System.Collections.Generic;
using UnityEngine;
using GoogleARCore;
public class ARCoreImageDetection : MonoBehaviour
{
// 需要检测的图像
public Texture2D detectionImage;
// 预制体
public GameObject prefab;
// 图像锚点
private DetectedImage detectedImage;
// 保存已经生成的预制体实例
private List<GameObject> instances = new List<GameObject>();
private void Start()
{
// 加载图像数据
byte[] imageBytes = detectionImage.EncodeToPNG();
// 创建图像配置
AugmentedImageDatabase augmentedImageDatabase = new AugmentedImageDatabase();
// 添加图像
augmentedImageDatabase.AddImage("detectionImage", new MutableAugmentedImageDatabaseEntry(imageBytes, "detectionImage"));
// 配置ARCore
ARCoreSessionConfig sessionConfig = new ARCoreSessionConfig();
sessionConfig.AugmentedImageDatabase = augmentedImageDatabase;
// 启动ARCore
ARCoreSession arCoreSession = FindObjectOfType<ARCoreSession>();
arCoreSession.SessionConfig = sessionConfig;
arCoreSession.enabled = true;
// 监听图像锚点事件
ARCoreSession.OnChooseCameraConfiguration += session => ChooseCameraConfiguration(session);
// 监听图像检测事件
AugmentedImageTrackingMethodHelper.OnAugmentedImageTrackingMethodChanged += method => AugmentedImageTrackingMethodChanged(method);
}
private void Update()
{
// 检测图像
Session.GetTrackables<DetectedImage>(detectedImages, TrackableQueryFilter.All);
// 遍历检测到的图像
foreach (var image in detectedImages)
{
// 如果检测到的是需要检测的图像
if (image.Name == "detectionImage")
{
// 如果该图像没有锚点
if (image.TrackingState == TrackingState.Tracking && detectedImage == null)
{
// 创建锚点
detectedImage = image;
ARCoreSession.CreateAnchor(detectedImage.CenterPose);
}
// 如果该图像有锚点
else if (image.TrackingState == TrackingState.Tracking && detectedImage != null && detectedImage.DatabaseIndex != image.DatabaseIndex)
{
// 销毁原有的预制体实例
foreach (var instance in instances)
{
Destroy(instance);
}
instances.Clear();
detectedImage = image;
ARCoreSession.CreateAnchor(detectedImage.CenterPose);
}
// 如果该图像丢失跟踪
else if (image.TrackingState == TrackingState.Stopped && detectedImage != null && detectedImage.DatabaseIndex == image.DatabaseIndex)
{
// 销毁预制体实例
foreach (var instance in instances)
{
Destroy(instance);
}
instances.Clear();
detectedImage = null;
}
}
}
}
private void ChooseCameraConfiguration(ARCoreSession arCoreSession)
{
// 选择相机配置
List<CameraConfig> cameraConfigs = new List<CameraConfig>(arCoreSession.GetSupportedCameraConfigs());
CameraConfig cameraConfig = cameraConfigs[0];
arCoreSession.RequestCameraConfiguration(cameraConfig);
}
private void AugmentedImageTrackingMethodChanged(AugmentedImageTrackingMethod trackingMethod)
{
// 设置图像跟踪方式
ARCoreSession arCoreSession = FindObjectOfType<ARCoreSession>();
arCoreSession.SessionConfig.SetAugmentedImageTrackingMethod(trackingMethod);
arCoreSession.enabled = false;
arCoreSession.enabled = true;
}
private void OnAnchorCreated(Anchor anchor)
{
// 将预制体添加到锚点上
GameObject instance = Instantiate(prefab, anchor.transform.position, anchor.transform.rotation);
instances.Add(instance);
}
}
```
代码中有注释,可以参考理解。
相关推荐
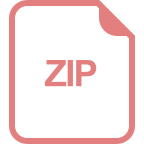
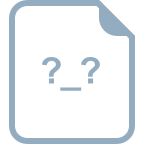
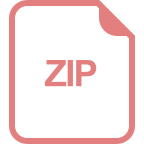














