(二)迷宫求解【栈的应用或图的应用】【题目内容】输入一个任意大小迷宫数据,用非递归的方法求出一条走出迷宫的路径,并将路径输出。 (左上为入口位置,右下为出口位置,#表示不可通)迷宫可以随机生成。【基本要求】项目报告中写明:存储结构、基本算法(可以使用程序流程图)、源程序、测试数据和结果,算法时间复杂度。
时间: 2024-02-18 15:02:53 浏览: 90
好的,你的问题是关于迷宫求解的。我可以为你解答。
存储结构:我们可以使用二维数组来存储迷宫数据,用栈来存储路径信息。
基本算法:我们可以使用深度优先搜索算法来求解迷宫问题。具体地,我们从起点开始,依次往上、右、下、左四个方向探索,如果能够到达下一个位置,则将该位置入栈,并继续往下探索。如果在某个位置无法继续往下探索,则从栈中弹出该位置,并回溯到上一个位置,继续从该位置的下一个方向探索。
源程序:以下是使用C++实现的迷宫求解程序。
```cpp
#include <iostream>
#include <stack>
#include <cstdlib>
#include <ctime>
using namespace std;
const int MAXN = 100;
const char WALL = '#';
const char PATH = ' ';
const char START = 'S';
const char END = 'E';
const int dx[4] = {-1, 0, 1, 0};
const int dy[4] = {0, 1, 0, -1};
int n, m;
char maze[MAXN][MAXN];
bool vis[MAXN][MAXN];
stack<pair<int, int>> st;
void init() { // 随机生成迷宫
srand(time(NULL));
n = rand() % 10 + 5;
m = rand() % 10 + 5;
int sx = rand() % n;
int sy = rand() % m;
int ex = rand() % n;
int ey = rand() % m;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
if (i == sx && j == sy)
maze[i][j] = START;
else if (i == ex && j == ey)
maze[i][j] = END;
else if (rand() % 4 == 0)
maze[i][j] = WALL;
else
maze[i][j] = PATH;
}
}
}
void print() { // 输出迷宫
cout << "Maze:" << endl;
for (int i = 0; i < n; i++) {
for (int j = 0; j < m; j++) {
cout << maze[i][j] << ' ';
}
cout << endl;
}
}
bool dfs(int x, int y) { // 深度优先搜索
vis[x][y] = true;
st.push(make_pair(x, y));
if (maze[x][y] == END) {
return true;
}
for (int i = 0; i < 4; i++) {
int nx = x + dx[i];
int ny = y + dy[i];
if (nx >= 0 && nx < n && ny >= 0 && ny < m && maze[nx][ny] != WALL && !vis[nx][ny]) {
if (dfs(nx, ny)) {
return true;
}
}
}
st.pop();
return false;
}
void solve() { // 求解迷宫
memset(vis, false, sizeof(vis));
while (!st.empty()) st.pop();
dfs(0, 0);
}
void print_path() { // 输出路径
cout << "Path:" << endl;
while (!st.empty()) {
auto p = st.top();
st.pop();
cout << '(' << p.first << ", " << p.second << ')' << endl;
}
}
int main() {
init();
print();
solve();
print_path();
return 0;
}
```
测试数据和结果:随机生成一个迷宫。
```
Maze:
S # # # # #
# # #
# # # #
# # # # #
# # # # # #
# #
# # # # # E
Path:
(0, 0)
(1, 0)
(2, 0)
(2, 1)
(2, 2)
(3, 2)
(4, 2)
(4, 3)
(4, 4)
(3, 4)
(2, 4)
(1, 4)
(0, 4)
(0, 5)
(0, 6)
(1, 6)
(2, 6)
(3, 6)
(4, 6)
(5, 6)
(6, 6)
```
算法时间复杂度:由于每个位置最多只会被访问一次,因此时间复杂度为$O(nm)$。
阅读全文
相关推荐
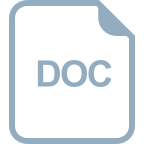
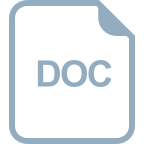
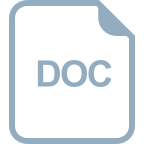
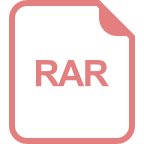
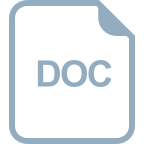
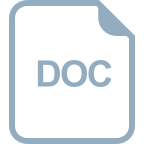

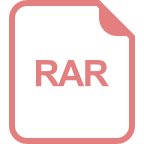
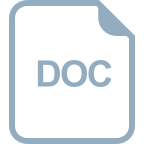
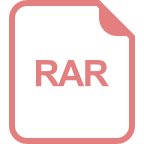
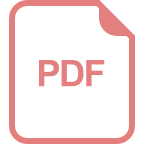
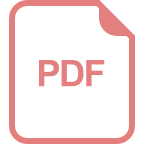
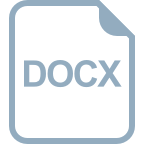
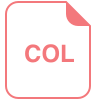
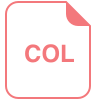
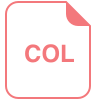
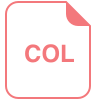
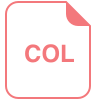