tf.keras.optimizers.Adam 中 learning_rate怎么随epoch更改
时间: 2024-05-05 08:21:43 浏览: 108
可以使用Keras的回调函数来实现在每个epoch结束时更改Adam优化器的learning_rate。具体步骤如下:
1. 创建一个回调函数类,继承自`tf.keras.callbacks.Callback`。在类中实现`on_epoch_end`方法,该方法会在每个epoch结束时被调用。
2. 在`on_epoch_end`方法中根据需要更新Adam优化器的learning_rate。
下面是一个示例代码:
```python
import tensorflow as tf
from tensorflow.keras.callbacks import Callback
class AdamLearningRateScheduler(Callback):
def __init__(self, initial_lr, epoch_decay):
super().__init__()
self.initial_lr = initial_lr
self.epoch_decay = epoch_decay
def on_epoch_end(self, epoch, logs=None):
lr = self.initial_lr / (1 + self.epoch_decay * epoch)
tf.keras.backend.set_value(self.model.optimizer.lr, lr)
print("Learning rate for epoch {} is {}".format(epoch+1, lr))
```
在上面的示例代码中,`AdamLearningRateScheduler`类继承自`tf.keras.callbacks.Callback`。它包含两个参数:`initial_lr`和`epoch_decay`,分别代表初始学习率和每个epoch的学习率下降率。
在`on_epoch_end`方法中,我们首先计算当前epoch的learning_rate,然后使用`tf.keras.backend.set_value`方法将新的学习率设置为Adam优化器的学习率,最后打印出当前epoch的学习率。
接下来,我们可以在Keras模型中使用这个回调函数。例如:
```python
from tensorflow.keras.optimizers import Adam
model = ... # 定义模型
initial_lr = 0.001
epoch_decay = 0.001
adam = Adam(learning_rate=initial_lr)
model.compile(optimizer=adam, ...)
lr_scheduler = AdamLearningRateScheduler(initial_lr, epoch_decay)
model.fit(..., callbacks=[lr_scheduler])
```
在上面的代码中,我们首先定义了一个Adam优化器,并将其作为模型的优化器。然后,我们创建了一个`AdamLearningRateScheduler`实例,并将其作为回调函数传递给`fit`方法。在训练过程中,每个epoch结束时,`AdamLearningRateScheduler`会根据当前epoch更新Adam优化器的学习率。
阅读全文
相关推荐
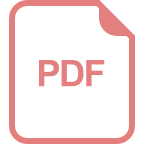
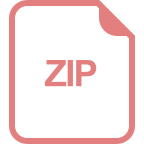








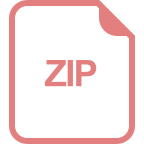
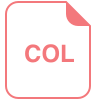
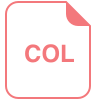
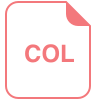
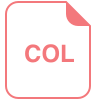
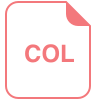
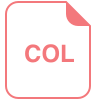

