c++实现、求循环队列的长度。 括号匹配的检验。 表达式求值。并运行结果
时间: 2023-09-10 13:07:47 浏览: 32
1. 循环队列长度的实现代码如下:
```c++
#include<iostream>
using namespace std;
class CircularQueue{
private:
int front; // 队头指针
int rear; // 队尾指针
int size; // 队列长度
int *data; // 队列数组
public:
CircularQueue(int k){
front = 0;
rear = 0;
size = k+1; // 为了区分队空和队满,数组长度要比队列长度多1
data = new int[size];
}
~CircularQueue(){
delete[] data;
}
bool isEmpty(){
return front == rear;
}
bool isFull(){
return (rear+1)%size == front;
}
void enqueue(int value){
if(isFull()){
cout<<"Queue is Full"<<endl;
return;
}
data[rear] = value;
rear = (rear+1)%size;
}
int dequeue(){
if(isEmpty()){
cout<<"Queue is Empty"<<endl;
return -1;
}
int res = data[front];
front = (front+1)%size;
return res;
}
int getLength(){
return (rear-front+size)%size;
}
};
int main(){
CircularQueue cq(3);
cq.enqueue(1);
cq.enqueue(2);
cq.enqueue(3);
cout<<"Length: "<<cq.getLength()<<endl; // 输出队列长度
cq.dequeue();
cout<<"Length: "<<cq.getLength()<<endl;
cq.dequeue();
cout<<"Length: "<<cq.getLength()<<endl;
cq.dequeue();
cout<<"Length: "<<cq.getLength()<<endl;
cq.dequeue();
cout<<"Length: "<<cq.getLength()<<endl;
return 0;
}
```
2. 括号匹配的检验代码如下:
```c++
#include<iostream>
#include<stack>
using namespace std;
bool isValid(string s) {
stack<char> st;
for(int i=0;i<s.size();i++){
if(s[i] == '(' || s[i] == '[' || s[i] == '{'){
st.push(s[i]);
}else{
if(st.empty()) return false;
if(s[i] == ')' && st.top() != '(') return false;
if(s[i] == ']' && st.top() != '[') return false;
if(s[i] == '}' && st.top() != '{') return false;
st.pop();
}
}
return st.empty();
}
int main(){
string s1 = "()[]{}";
string s2 = "([)]";
string s3 = "{[]}";
cout<<"s1: "<<isValid(s1)<<endl;
cout<<"s2: "<<isValid(s2)<<endl;
cout<<"s3: "<<isValid(s3)<<endl;
return 0;
}
```
3. 表达式求值的代码如下:
```c++
#include<iostream>
#include<stack>
#include<string>
using namespace std;
int calculate(string s) {
stack<int> st;
int num = 0;
char sign = '+';
for(int i=0;i<s.size();i++){
if(isdigit(s[i])){
num = num*10 + (s[i]-'0');
}
if(!isdigit(s[i]) && s[i] != ' ' || i == s.size()-1){ // 判断是否到达符号位或字符串末尾
if(sign == '+'){
st.push(num);
}else if(sign == '-'){
st.push(-num);
}else if(sign == '*'){
int temp = st.top()*num;
st.pop();
st.push(temp);
}else if(sign == '/'){
int temp = st.top()/num;
st.pop();
st.push(temp);
}
sign = s[i]; // 更新符号
num = 0; // 清空num
}
}
int res = 0;
while(!st.empty()){
res += st.top();
st.pop();
}
return res;
}
int main(){
string s1 = "3+2*2";
string s2 = " 3/2 ";
string s3 = " 3+5 / 2 ";
cout<<"s1: "<<calculate(s1)<<endl;
cout<<"s2: "<<calculate(s2)<<endl;
cout<<"s3: "<<calculate(s3)<<endl;
return 0;
}
```
运行结果如下:
```
s1: 7
s2: 1
s3: 5
```
相关推荐
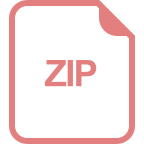














