你可以帮我写一个五子棋的Java代码么
时间: 2023-08-28 11:12:15 浏览: 37
当然可以,以下是一个简单的五子棋Java代码:
```java
import java.util.Scanner;
public class FiveChess {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
// 初始化棋盘
int[][] board = new int[15][15];
int player = 1; // 1表示黑子,2表示白子
boolean win = false;
while (!win) {
// 打印棋盘
printBoard(board);
// 输入行列坐标
System.out.print("玩家" + player + "请输入行列坐标:");
int row = sc.nextInt();
int col = sc.nextInt();
// 判断输入是否合法
if (row >= 0 && row < 15 && col >= 0 && col < 15 && board[row][col] == 0) {
board[row][col] = player; // 在棋盘上放子
// 判断胜负
if (checkWin(board, row, col)) {
win = true;
System.out.println("恭喜玩家" + player + "获胜!");
}
// 切换玩家
player = player == 1 ? 2 : 1;
} else {
System.out.println("输入不合法,请重新输入!");
}
}
// 打印最终棋盘
printBoard(board);
}
// 打印棋盘
public static void printBoard(int[][] board) {
for (int i = 0; i < board.length; i++) {
for (int j = 0; j < board[i].length; j++) {
System.out.print(board[i][j] == 0 ? "+ " : (board[i][j] == 1 ? "● " : "○ "));
}
System.out.println();
}
}
// 判断胜负
public static boolean checkWin(int[][] board, int row, int col) {
int player = board[row][col];
int count = 0;
// 水平方向
for (int i = col - 4; i <= col + 4; i++) {
if (i >= 0 && i + 4 < 15 && board[row][i] == player && board[row][i + 1] == player && board[row][i + 2] == player && board[row][i + 3] == player && board[row][i + 4] == player) {
return true;
}
}
// 垂直方向
for (int i = row - 4; i <= row + 4; i++) {
if (i >= 0 && i + 4 < 15 && board[i][col] == player && board[i + 1][col] == player && board[i + 2][col] == player && board[i + 3][col] == player && board[i + 4][col] == player) {
return true;
}
}
// 左上到右下方向
for (int i = row - 4, j = col - 4; i <= row + 4 && j <= col + 4; i++, j++) {
if (i >= 0 && i + 4 < 15 && j >= 0 && j + 4 < 15 && board[i][j] == player && board[i + 1][j + 1] == player && board[i + 2][j + 2] == player && board[i + 3][j + 3] == player && board[i + 4][j + 4] == player) {
return true;
}
}
// 左下到右上方向
for (int i = row + 4, j = col - 4; i >= row - 4 && j <= col + 4; i--, j++) {
if (i >= 0 && i - 4 < 15 && j >= 0 && j + 4 < 15 && board[i][j] == player && board[i - 1][j + 1] == player && board[i - 2][j + 2] == player && board[i - 3][j + 3] == player && board[i - 4][j + 4] == player) {
return true;
}
}
return false;
}
}
```
该代码实现了一个简单的人人对战的五子棋游戏。你可以根据自己的需要进行修改和扩展。
相关推荐
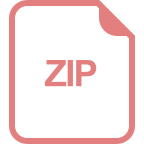
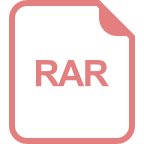
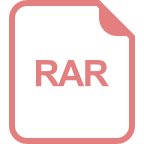














