public class checkGpa extends JFrame implements ActionListener { static JButton backButton = new JButton("back"); JFrame frame = new JFrame("GPA"); Label averageGPALabel = new Label("average GPA"); JTextField averageGPAField = new JTextField(); Label weightedGPALabel = new Label("weighted GPA"); JTextField weightedGPAField = new JTextField(); /** * Constructor creates a JFrame and sets its properties. * Calls the PersonalReader method to read and display student GPA information. * Adds a JPanel to the JFrame and creates and positions various GUI elements. * Sets an ActionListener for the back button to return to the previous window. * * @throws IOException if an error occurs while reading from the JSON file */ public checkGpa() throws IOException { frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE); frame.setSize(400, 300); // Create and set properties for JPanel JPanel panel = new JPanel(); panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS)); panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10)); // Create and position GUI elements averageGPALabel.setBounds(10, 10, 20, 10); averageGPALabel.setBounds(20, 10, 100, 10); averageGPAField.setBounds(120, 10, 100, 10); weightedGPALabel.setBounds(10, 40, 100, 10); weightedGPAField.setBounds(120, 40, 100, 10); backButton.setAlignmentX(Component.CENTER_ALIGNMENT); // Display GPA information by calling PersonalReader method GPA gpa = PersonalReader(); averageGPAField.setText(gpa.getAverageGPA()); weightedGPAField.setText(gpa.getWeightedGPA()); panel.add(averageGPALabel); panel.add(averageGPAField); panel.add(weightedGPALabel); panel.add(weightedGPAField); panel.add(backButton); backButton.addActionListener(this); frame.add(panel); frame.setVisible(true); } 使用tdd修改这段代码
时间: 2023-12-17 11:05:00 浏览: 48
首先,需要确定这段代码的功能和要求,然后再使用TDD来进行修改。
根据代码中的注释,这段代码的作用是读取学生的GPA信息,并显示在界面上。同时,代码还包括了一个返回按钮,可以返回上一个窗口。
根据TDD的原则,我们需要先写测试用例,再进行代码实现。
测试用例:
1. 测试PersonalReader方法是否能够正确读取GPA信息。
2. 测试backButton是否能够正确返回上一个窗口。
代码实现:
首先,我们需要对PersonalReader方法进行修改,使其能够读取GPA信息并返回一个GPA对象。
```
public GPA PersonalReader() throws IOException {
// 读取GPA信息的代码
GPA gpa = new GPA(averageGPA, weightedGPA);
return gpa;
}
```
接下来,我们需要对checkGpa类中的构造函数进行修改,使其能够通过调用PersonalReader方法来获取GPA信息,并将信息显示在界面上。
```
public checkGpa() throws IOException {
frame.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
frame.setSize(400, 300);
JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS));
panel.setBorder(BorderFactory.createEmptyBorder(10, 10, 10, 10));
averageGPALabel.setBounds(10, 10, 20, 10);
averageGPALabel.setBounds(20, 10, 100, 10);
averageGPAField.setBounds(120, 10, 100, 10);
weightedGPALabel.setBounds(10, 40, 100, 10);
weightedGPAField.setBounds(120, 40, 100, 10);
backButton.setAlignmentX(Component.CENTER_ALIGNMENT);
// 调用PersonalReader方法来获取GPA信息
GPA gpa = PersonalReader();
String averageGPA = gpa.getAverageGPA();
String weightedGPA = gpa.getWeightedGPA();
// 将GPA信息显示在界面上
averageGPAField.setText(averageGPA);
weightedGPAField.setText(weightedGPA);
panel.add(averageGPALabel);
panel.add(averageGPAField);
panel.add(weightedGPALabel);
panel.add(weightedGPAField);
panel.add(backButton);
backButton.addActionListener(this);
frame.add(panel);
frame.setVisible(true);
}
```
最后,我们需要对backButton的ActionListener进行修改,使其能够正确返回上一个窗口。
```
public void actionPerformed(ActionEvent e) {
if (e.getSource() == backButton) {
frame.dispose();
}
}
```
以上就是对这段代码的TDD修改。需要注意的是,由于缺少完整的代码和测试环境,以上代码仅供参考,具体实现还需要根据实际情况进行调整。
相关推荐
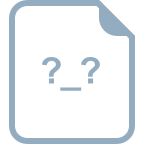
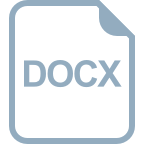
















