if (agvs[i].battery_ <100){ std::thread charge_thread([&]() { agv_charge(i); }); charge_thread.detach(); },增加代碼:黨綫程結束的時候,運行moveAgvs(i);
时间: 2024-02-14 16:06:27 浏览: 75
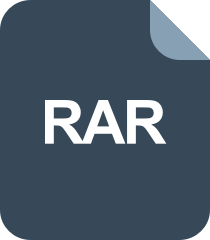
AGVS.rar_AGVS调度_gulf5j4_scheduling AGV_wpf agv调度系统_调度控制

如果你想在新线程结束时运行 `moveAgvs(i)`,可以将代码修改为:
```
if (agvs[i].battery_ < 100) {
auto i_copy = std::move(agvs[i]);
std::thread charge_thread([i_copy]() {
agv_charge(i_copy);
// 在线程结束时运行moveAgvs(i)
moveAgvs(i_copy);
});
charge_thread.detach();
}
```
这里先将 `agvs[i]` 移动到一个新的变量 `i_copy` 中,然后在新线程中使用 `i_copy`,确保在线程中可以访问到它。在线程结束时,先运行 `moveAgvs(i_copy)`,然后再运行 `move(std::move(i_copy))`,将 `i_copy` 移动到别处。
阅读全文
相关推荐
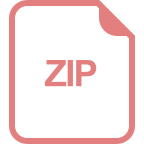











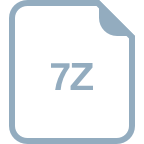
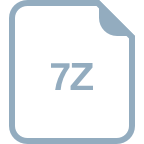