定义一个基类Shape,在此基础上派生出Rectangle和Circle,二者都有一个GetArea()函数计算对象面积。使用Rectangle类创建一个派生类Square。
时间: 2024-05-08 20:19:56 浏览: 15
好的,根据您的要求,我可以参考以下代码实现:
```
class Shape:
def getArea(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def getArea(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def getArea(self):
return 3.14 * (self.radius ** 2)
class Square(Rectangle):
def __init__(self, length):
super().__init__(length, length)
```
在上面的代码中,我们先定义了一个基类Shape,其中有一个抽象方法getArea()。接着,我们派生出了Rectangle和Circle两个具体的子类,并实现了它们的getArea()方法。最后,我们又派生出了一个Square子类,它继承了Rectangle类,并重写了构造函数以保证它的长宽相等。这样,我们就可以通过Square类计算正方形面积了。
相关问题
定义一个基类Shape,在此基础上派生出Rectangle和Circle类,二者都有Getarea()计算对象面积
下面是一个示例代码,实现了Shape基类以及Rectangle和Circle派生类,并且每个类都有GetArea()函数来计算对象面积:
```c++
#include <iostream>
using namespace std;
class Shape
{
public:
virtual double GetArea() = 0;
};
class Rectangle : public Shape
{
private:
double length;
double width;
public:
Rectangle(double l, double w) : length(l), width(w) {}
double GetArea() { return length * width; }
};
class Circle : public Shape
{
private:
double radius;
public:
Circle(double r) : radius(r) {}
double GetArea() { return 3.1415926 * radius * radius; }
};
int main()
{
Shape* shape1 = new Rectangle(5, 10);
Shape* shape2 = new Circle(3);
cout << "Rectangle area: " << shape1->GetArea() << endl;
cout << "Circle area: " << shape2->GetArea() << endl;
delete shape1;
delete shape2;
return 0;
}
```
在上面的代码中,Shape是一个纯虚基类,它提供了一个GetArea()函数但没有实现。Rectangle和Circle派生类都实现了这个函数,并且在main函数里我们创建了一个Rectangle对象和一个Circle对象,并分别调用它们的GetArea()函数来计算它们的面积。注意,我们使用了Shape指针来指向Rectangle和Circle对象,这是因为Shape是一个基类,它的指针可以指向任何派生类对象。
定义一个基类Shape,在此基础上派生出Rectangle和Circle类,二者都有GetArea()计算对象的面积。
下面是代码实现:
```python
class Shape:
def __init__(self):
pass
def GetArea(self):
pass
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def GetArea(self):
return self.width * self.height
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def GetArea(self):
return 3.14 * self.radius ** 2
```
在这里,我们定义了一个基类 `Shape`,并且把 `Rectangle` 和 `Circle` 派生出来。`Shape` 类中只有一个空的初始化函数和一个 `GetArea` 方法,没有具体实现。而 `Rectangle` 和 `Circle` 类则分别实现了自己的构造函数和 `GetArea` 方法。
`Rectangle` 类中,我们定义了一个 `width` 和 `height` 属性,构造函数中传入这两个参数,并且在 `GetArea` 方法中返回面积。同理,`Circle` 类中,我们定义了一个 `radius` 属性,构造函数中传入这个参数,并且在 `GetArea` 方法中返回面积。
相关推荐
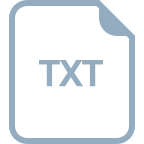
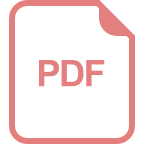
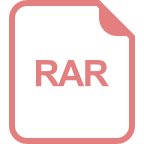











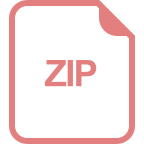
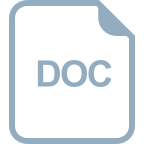