用python写一段建立一阶直线倒立摆模型的代码
时间: 2023-06-03 19:04:28 浏览: 93
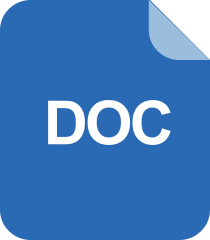
基于PID一阶倒立摆控制与仿真.doc

当然,我可以为您提供关于建立一阶直线倒立摆模型的 python 。
首先,您需要导入相应的库:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
```
然后,定义相关的参数:
```python
# 定义参数
g = 9.81 # 重力加速度
l = 2.0 # 杆子长度
m = 1.0 # 杆子质量
b = 0.0 # 摩擦系数
```
接着,定义运动方程:
```python
# 定义运动方程
def inverted_pendulum_equations(state, t):
theta, theta_dot = state # 状态向量
theta_dot_dot = (-(g/l)*np.sin(theta) - b*theta_dot)/(m*l**2)
return [theta_dot, theta_dot_dot]
```
然后,设置初始状态并求解:
```python
# 设置初始状态
theta0, theta_dot0 = 0.1, 0.0
state0 = [theta0, theta_dot0]
# 设置时间区间
t_start, t_end, dt = 0.0, 30.0, 0.01
t = np.arange(t_start, t_end+dt, dt)
# 求解方程
state = odeint(inverted_pendulum_equations, state0, t)
```
最后,绘制出摆的运动轨迹:
```python
# 绘制摆的运动轨迹
theta = state[:, 0] # 杆子的角度
x = l * np.sin(theta) # 杆子的横坐标
y = -l * np.cos(theta) # 杆子的纵坐标
plt.plot(t, y, 'b')
plt.title('Inverted Pendulum Motion')
plt.xlabel('Time (s)')
plt.ylabel('Distance from the Bottom (m)')
plt.show()
```
这样,您就可以建立一阶直线倒立摆模型的 python 代码了。
阅读全文
相关推荐
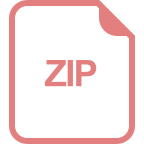
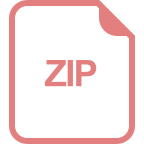













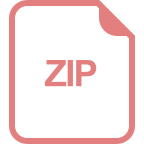
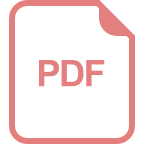