运用c++编写对于给定的二叉树,输出其先序序列、中序序列、后序序列并输出叶子结点数。 输入格式: 二叉树的层次序列。 提示:一棵二叉树的层次序列是一个字符串,若字符是‘#’,表示该二叉树是空树,否则该字符是相应结点的数据元素。 输出格式: 若是非空二叉树,则输出四行内容 第一行是二叉树的先序遍历序列; 第二行是二叉树的中序遍历序列; 第三行是二叉树的后序遍历序列; 第四行是叶子结点数; 若是空二叉树 只需输出叶子数0
时间: 2023-08-10 10:43:54 浏览: 159
好的,下面是代码实现:
```
#include<iostream>
#include<queue>
#include<stack>
using namespace std;
class BinaryTreeNode {
public:
int val;
BinaryTreeNode* left;
BinaryTreeNode* right;
BinaryTreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
void createBinaryTree(BinaryTreeNode*& root, string s) {
if (s.length() == 0 || s[0] == '#') {
root = nullptr;
return;
}
int val = 0, i = 0;
while (s[i] != ',' && i < s.length()) {
val = val * 10 + s[i] - '0';
i++;
}
root = new BinaryTreeNode(val);
if (i == s.length()) return;
int j = i + 1, k = j;
while (k < s.length()) {
if (s[k] == ',') break;
k++;
}
createBinaryTree(root->left, s.substr(j, k - j));
createBinaryTree(root->right, s.substr(k + 1));
}
// 先序遍历
void preorderTraversal(BinaryTreeNode* root) {
if (root == nullptr) return;
cout << root->val << " ";
preorderTraversal(root->left);
preorderTraversal(root->right);
}
// 中序遍历
void inorderTraversal(BinaryTreeNode* root) {
if (root == nullptr) return;
inorderTraversal(root->left);
cout << root->val << " ";
inorderTraversal(root->right);
}
// 后序遍历
void postorderTraversal(BinaryTreeNode* root) {
if (root == nullptr) return;
postorderTraversal(root->left);
postorderTraversal(root->right);
cout << root->val << " ";
}
// 计算叶子结点数
int countLeafNodes(BinaryTreeNode* root) {
if (root == nullptr) return 0;
if (root->left == nullptr && root->right == nullptr) return 1;
return countLeafNodes(root->left) + countLeafNodes(root->right);
}
int main() {
string s;
cin >> s;
BinaryTreeNode* root;
createBinaryTree(root, s);
if (root == nullptr) {
cout << "叶子结点数:0" << endl;
return 0;
}
cout << "先序遍历:" << endl;
preorderTraversal(root);
cout << endl << "中序遍历:" << endl;
inorderTraversal(root);
cout << endl << "后序遍历:" << endl;
postorderTraversal(root);
cout << endl << "叶子结点数:" << countLeafNodes(root) << endl;
return 0;
}
```
使用方法:
- 输入二叉树的层次序列,其中 "#" 表示空节点。
- 输出先序序列、中序序列、后序序列,以及叶子结点数。
希望能够帮到你!
阅读全文
相关推荐

















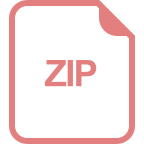