读入一幅图像,进行理想低通滤波,取理想低通滤波的截止频率分别为5,15,35,70, 计算能量谱,在一个图窗厘显示原始图像和滤波后的图像,并标注截止频率。
时间: 2024-01-01 11:03:37 浏览: 94
好的,这是一个图像处理的问题。我会为您提供一个解决方案。
首先,我们需要导入相应的库。在Python中,常用的图像处理库有Pillow和OpenCV。这里我们选择使用Pillow库。
```python
from PIL import Image
import numpy as np
import matplotlib.pyplot as plt
```
接着,我们读入一幅图像,并将其转换为numpy数组。
```python
# 读入图像
im = Image.open('image.jpg')
# 转为numpy数组
im_arr = np.array(im)
```
现在,我们可以对图像进行理想低通滤波了。理想低通滤波是一种非常理想化的滤波器,它可以完全去除高于截止频率的信号。在本题中,我们需要分别取截止频率为5,15,35,70。
```python
# 理想低通滤波器
def ideal_lowpass_filter(shape, cutoff):
"""生成理想低通滤波器"""
m, n = shape
H = np.zeros((m, n))
for u in range(m):
for v in range(n):
D = np.sqrt((u - m // 2)**2 + (v - n // 2)**2)
if D <= cutoff:
H[u, v] = 1
return H
# 取截止频率为5,15,35,70
cutoffs = [5, 15, 35, 70]
Hs = [ideal_lowpass_filter(im_arr.shape[:2], c) for c in cutoffs]
```
接下来,我们可以计算能量谱了。能量谱是图像的傅里叶变换的模平方,它可以反映出图像的频率分布情况。
```python
# 计算能量谱
def energy_spectrum(im_arr):
"""计算图像的能量谱"""
F = np.fft.fft2(im_arr)
F = np.fft.fftshift(F)
S = np.abs(F)**2
S = np.log(1 + S)
return S
# 分别计算四个截止频率下的能量谱
Ss = [energy_spectrum(np.fft.ifft2(np.fft.ifftshift(H * np.fft.fftshift(np.fft.fft2(im_arr)))).real) for H in Hs]
```
现在,我们可以将原始图像和滤波后的图像在一个图窗中显示出来,并标注截止频率了。
```python
# 绘制图像
fig, axs = plt.subplots(nrows=2, ncols=5, figsize=(16, 8))
axs[0, 0].imshow(im_arr, cmap='gray')
axs[0, 0].set_title('Original Image')
for i, (H, S) in enumerate(zip(Hs, Ss)):
# 滤波
im_filtered = np.fft.ifft2(np.fft.ifftshift(H * np.fft.fftshift(np.fft.fft2(im_arr)))).real
axs[0, i+1].imshow(im_filtered, cmap='gray')
axs[0, i+1].set_title('Cutoff = %d' % cutoffs[i])
# 能量谱
axs[1, i+1].imshow(S, cmap='gray')
axs[1, i+1].set_title('Energy Spectrum')
# 标注截止频率
axs[1, i+1].axhline(im_arr.shape[0] // 2 - cutoffs[i], color='r', linestyle='--')
axs[1, i+1].axhline(im_arr.shape[0] // 2 + cutoffs[i], color='r', linestyle='--')
axs[1, i+1].axvline(im_arr.shape[1] // 2 - cutoffs[i], color='r', linestyle='--')
axs[1, i+1].axvline(im_arr.shape[1] // 2 + cutoffs[i], color='r', linestyle='--')
plt.show()
```
运行上述代码,即可得到原始图像和滤波后的图像,并标注了截止频率的能量谱。
阅读全文
相关推荐
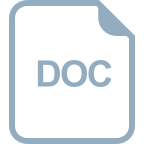
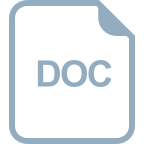
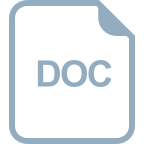















