模拟退火的电子锯材料排版C#代码
时间: 2023-10-19 10:16:36 浏览: 46
以下是一个简单的模拟退火算法的电子锯材料排版示例,使用了C#编程语言:
```csharp
using System;
using System.Collections.Generic;
namespace SimulatedAnnealing
{
class Program
{
static void Main(string[] args)
{
int boardWidth = 100; // 板的宽度
int boardHeight = 100; // 板的高度
int numPieces = 10; // 需要排版的零件数
List<Piece> pieces = new List<Piece>(); // 存储所有零件
// 初始化所有零件
for (int i = 0; i < numPieces; i++)
{
int width = new Random().Next(10, 20); // 随机生成零件的宽度
int height = new Random().Next(10, 20); // 随机生成零件的高度
pieces.Add(new Piece(width, height));
}
// 运行模拟退火算法
Layout layout = SimulatedAnnealing(boardWidth, boardHeight, pieces);
// 输出结果
Console.WriteLine("Final layout:");
Console.WriteLine(layout.ToString());
Console.ReadLine();
}
static Layout SimulatedAnnealing(int boardWidth, int boardHeight, List<Piece> pieces)
{
double temperature = 1000; // 初始温度
double coolingRate = 0.03; // 冷却速率
double minTemperature = 0.1; // 最小温度
Layout currentLayout = new Layout(boardWidth, boardHeight); // 当前布局
Layout bestLayout = new Layout(boardWidth, boardHeight); // 最优布局
bestLayout.FitPieces(pieces); // 将所有零件放到最优布局中
while (temperature > minTemperature)
{
Layout newLayout = currentLayout.Clone(); // 克隆当前布局
newLayout.Mutate(); // 随机移动一个零件
double currentEnergy = currentLayout.CalculateEnergy(pieces); // 当前能量
double newEnergy = newLayout.CalculateEnergy(pieces); // 新能量
if (newEnergy < currentEnergy || Math.Exp((currentEnergy - newEnergy) / temperature) > new Random().NextDouble())
{
currentLayout = newLayout;
if (currentEnergy < bestLayout.CalculateEnergy(pieces))
{
bestLayout = currentLayout.Clone();
}
}
temperature *= 1 - coolingRate; // 降低温度
}
return bestLayout;
}
}
// 零件类
class Piece
{
public int Width { get; set; } // 宽度
public int Height { get; set; } // 高度
public int X { get; set; } // x坐标
public int Y { get; set; } // y坐标
public Piece(int width, int height)
{
Width = width;
Height = height;
}
}
// 布局类
class Layout
{
public int Width { get; set; } // 宽度
public int Height { get; set; } // 高度
public List<Piece> Pieces { get; set; } // 零件列表
public Layout(int width, int height)
{
Width = width;
Height = height;
Pieces = new List<Piece>();
}
// 将所有零件放到布局中
public void FitPieces(List<Piece> pieces)
{
int x = 0;
int y = 0;
foreach (Piece piece in pieces)
{
piece.X = x;
piece.Y = y;
Pieces.Add(piece);
x += piece.Width;
if (x >= Width)
{
x = 0;
y += piece.Height;
}
}
}
// 计算能量
public double CalculateEnergy(List<Piece> pieces)
{
double energy = 0;
foreach (Piece piece in Pieces)
{
if (piece.X + piece.Width > Width || piece.Y + piece.Height > Height)
{
energy += 100000; // 超出边界的能量惩罚
}
foreach (Piece otherPiece in Pieces)
{
if (piece != otherPiece)
{
int dx = Math.Abs(piece.X - otherPiece.X);
int dy = Math.Abs(piece.Y - otherPiece.Y);
if (dx < piece.Width && dy < piece.Height)
{
energy += 100000; // 重叠的能量惩罚
}
}
}
}
return energy;
}
// 克隆布局
public Layout Clone()
{
Layout newLayout = new Layout(Width, Height);
foreach (Piece piece in Pieces)
{
newLayout.Pieces.Add(new Piece(piece.Width, piece.Height)
{
X = piece.X,
Y = piece.Y
});
}
return newLayout;
}
// 随机移动一个零件
public void Mutate()
{
Piece piece = Pieces[new Random().Next(Pieces.Count)];
int dx = new Random().Next(-10, 10);
int dy = new Random().Next(-10, 10);
piece.X += dx;
piece.Y += dy;
}
// 输出布局
public override string ToString()
{
string output = "";
for (int y = 0; y < Height; y++)
{
for (int x = 0; x < Width; x++)
{
bool foundPiece = false;
foreach (Piece piece in Pieces)
{
if (x >= piece.X && x < piece.X + piece.Width && y >= piece.Y && y < piece.Y + piece.Height)
{
output += "X";
foundPiece = true;
break;
}
}
if (!foundPiece)
{
output += ".";
}
}
output += "\n";
}
return output;
}
}
}
```
该示例使用了模拟退火算法来解决电子锯材料排版问题,它的实现过程如下:
1. 定义了一个`Piece`类来表示零件,包含宽度、高度、x坐标和y坐标等属性。
2. 定义了一个`Layout`类来表示布局,包含宽度、高度和零件列表等属性,以及一些方法来计算能量、克隆布局、随机移动一个零件等。
3. 在`Main`方法中创建了一些随机大小的零件,并调用`SimulatedAnnealing`方法来运行模拟退火算法,最后输出最优布局。
在`SimulatedAnnealing`方法中,使用了一个循环来不断降低温度,直到达到最小温度为止。在每个温度下,随机移动一个零件,并计算当前能量和新能量。如果新能量更低,则接受新布局;否则,根据概率接受新布局。在整个模拟退火算法的过程中,记录最优布局,并返回它。
最后,在`Layout`类中,定义了一个`ToString`方法,以便将布局输出为字符串,方便查看。
相关推荐
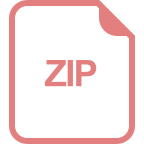
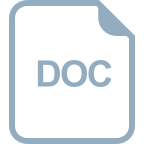














