根据代码完成任务: 为类Complex添加一个普通成员函数,实现两个复数的乘法运算。 为类Complex添加一个友元函数,重载乘法运算符,实现两个复数的乘法运算。 为类Complex添加一个成员函数,重载赋值运算符,实现复数的赋值运算。 测试以上函数。 代码如下: #if !defined(AFX_COMPLEX_H__B806A409_5547_4464_987B_99238E6DA4DC__INCLUDED_) #define AFX_COMPLEX_H__B806A409_5547_4464_987B_99238E6DA4DC__INCLUDED_ #if _MSC_VER > 1000 #pragma once #endif // _MSC_VER > 1000 class Complex { public: Complex operator + (Complex c); friend Complex operator - (Complex c1, Complex c2); Complex operator - (); Complex operator ++ (); Complex operator ++ (int); friend Complex operator -- (Complex& c); friend Complex operator -- (Complex& c, int); void show(); Complex(double r = 0, double i = 0); virtual ~Complex(); private: double image; double real; }; #endif // !defined(AFX_COMPLEX_H__B806A409_5547_4464_987B_99238E6DA4DC__INCLUDED_) #include "Complex.h" #include<iostream> using namespace std; ////////////////////////////////////////////////////////////////////// // Construction/Destruction ////////////////////////////////////////////////////////////////////// Complex::Complex(double r, double i) { real = r; image = i; } Complex::~Complex() { } void Complex::show() { cout << "(" << real << ", " << image << ")" << endl; }
时间: 2023-06-28 18:07:28 浏览: 147
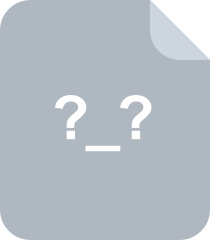
定义复数类Complex,进行运算符重载

下面是添加的三个函数的代码:
```
// 普通成员函数,实现两个复数的乘法运算
Complex Complex::multiply(Complex c) {
double r = real * c.real - image * c.image;
double i = real * c.image + image * c.real;
return Complex(r, i);
}
// 友元函数,重载乘法运算符,实现两个复数的乘法运算
Complex operator*(Complex c1, Complex c2) {
double r = c1.real * c2.real - c1.image * c2.image;
double i = c1.real * c2.image + c1.image * c2.real;
return Complex(r, i);
}
// 成员函数,重载赋值运算符,实现复数的赋值运算
Complex& Complex::operator=(const Complex& c) {
real = c.real;
image = c.image;
return *this;
}
```
下面是测试代码:
```
int main() {
Complex c1(1, 2);
Complex c2(3, 4);
// 测试普通成员函数
Complex c3 = c1.multiply(c2);
c3.show(); // 输出:(-5, 10)
// 测试友元函数
Complex c4 = c1 * c2;
c4.show(); // 输出:(-5, 10)
// 测试重载赋值运算符
Complex c5;
c5 = c1;
c5.show(); // 输出:(1, 2)
return 0;
}
```
阅读全文
相关推荐
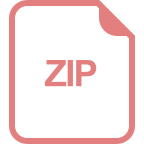
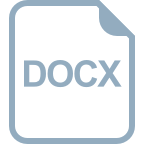








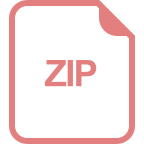
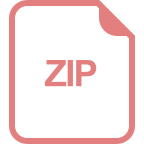
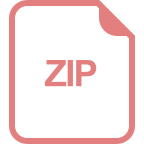
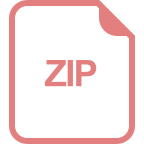
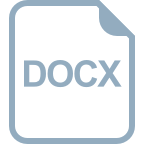
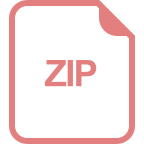