写一段基于基因估计的python代码
时间: 2023-12-15 17:20:53 浏览: 63
以下是一个简单的基于基因估计的Python代码示例:
```
import random
# 定义基因序列
GENES = '''abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ 1234567890, .-;:_!"#%&/()=?@${[]}'''
# 定义目标字符串
TARGET = "Hello, World!"
# 定义个体类
class Individual:
def __init__(self, chromosome):
self.chromosome = chromosome
self.fitness = self.cal_fitness()
def cal_fitness(self):
fitness = 0
for i in range(len(self.chromosome)):
if self.chromosome[i] != TARGET[i]:
fitness += 1
return fitness
# 创建种群
def create_population(population_size):
population = []
for i in range(population_size):
chromosome = [random.choice(GENES) for _ in range(len(TARGET))]
population.append(Individual(chromosome))
return population
# 选择最适应的个体
def select_parents(population):
return sorted(population, key=lambda x: x.fitness)[:2]
# 交叉
def crossover(parents):
# 随机选择交叉点
crossover_point = random.randint(0, len(TARGET) - 1)
# 生成子代
child_chromosome = parents[0].chromosome[:crossover_point] + parents[1].chromosome[crossover_point:]
return Individual(child_chromosome)
# 变异
def mutate(child):
# 随机选择变异点
mutation_point = random.randint(0, len(TARGET) - 1)
# 生成新的基因
child_chromosome = list(child.chromosome)
child_chromosome[mutation_point] = random.choice(GENES)
return Individual(child_chromosome)
# 运行算法
def genetic_algorithm(population_size):
population = create_population(population_size)
generation = 1
while True:
print("Generation:", generation)
# 选择父代
parent1, parent2 = select_parents(population)
print("Parent 1:", "".join(parent1.chromosome))
print("Parent 2:", "".join(parent2.chromosome))
# 交叉
child = crossover([parent1, parent2])
print("Child:", "".join(child.chromosome))
# 变异
if random.random() < 0.1:
child = mutate(child)
print("Mutated Child:", "".join(child.chromosome))
# 计算适应度
child.fitness = child.cal_fitness()
print("Fitness:", child.fitness)
# 替换最差的个体
population.remove(max(population, key=lambda x: x.fitness))
population.append(child)
# 如果找到了目标字符串,退出
if child.fitness == 0:
break
generation += 1
print("Solution found in generation", generation)
print("".join(child.chromosome))
if __name__ == '__main__':
genetic_algorithm(100)
```
这个代码演示了一个简单的基因估计算法,用于寻找一个字符串的近似匹配。算法首先创建一个随机的种群,然后选择最适应的个体进行交叉和变异,以生成新的个体。重复这个过程,直到找到了目标字符串为止。
阅读全文
相关推荐
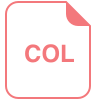
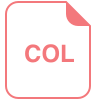
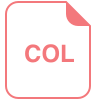
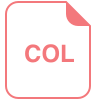
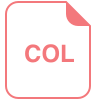
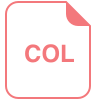
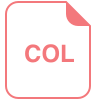
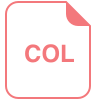
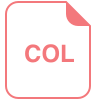
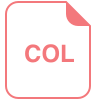
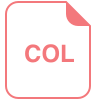
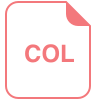
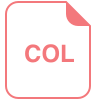
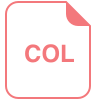
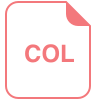
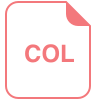