帮我写一段切比雪夫做评估函函数、加入惩罚函数得A*算法代码
时间: 2023-12-23 14:02:43 浏览: 71
好的,以下是一个简单的 A* 算法的实现,其中使用切比雪夫距离作为评估函数,并加入了惩罚函数:
```python
import heapq
from typing import List, Tuple
# 定义地图节点类
class Node:
def __init__(self, x: int, y: int, g: int = 0, h: int = 0, parent: 'Node' = None):
self.x = x
self.y = y
self.g = g # 起点到当前节点的距离
self.h = h # 当前节点到终点的估计距离
self.parent = parent
def f(self):
return self.g + self.h
# 计算切比雪夫距离
def chebyshev_distance(a: Tuple[int, int], b: Tuple[int, int]) -> int:
return max(abs(a[0] - b[0]), abs(a[1] - b[1]))
# 定义惩罚函数
def penalty_function(node: Node, obstacles: List[Tuple[int, int]]) -> int:
penalty = 0
for obstacle in obstacles:
if abs(node.x - obstacle[0]) <= 1 and abs(node.y - obstacle[1]) <= 1:
penalty += 100
return penalty
# A*算法实现
def astar(start: Tuple[int, int], end: Tuple[int, int], obstacles: List[Tuple[int, int]]) -> List[Tuple[int, int]]:
open_list = []
closed_list = []
start_node = Node(start[0], start[1], 0, chebyshev_distance(start, end) + penalty_function(Node(start[0], start[1]), obstacles))
heapq.heappush(open_list, (start_node.f(), start_node))
while open_list:
current_node = heapq.heappop(open_list)[1]
if (current_node.x, current_node.y) == end:
path = []
while current_node:
path.append((current_node.x, current_node.y))
current_node = current_node.parent
return path[::-1]
closed_list.append((current_node.x, current_node.y))
# 遍历当前节点的相邻节点
for dx, dy in [(1, 0), (-1, 0), (0, 1), (0, -1)]:
x, y = current_node.x + dx, current_node.y + dy
# 判断节点是否在地图内
if x < 0 or x >= len(obstacles) or y < 0 or y >= len(obstacles[0]):
continue
# 判断节点是否为障碍物或者已经访问过
if (x, y) in obstacles or (x, y) in closed_list:
continue
# 计算节点代价
g = current_node.g + 1
h = chebyshev_distance((x, y), end)
f = g + h + penalty_function(Node(x, y), obstacles)
node = Node(x, y, g, h, current_node)
# 将节点加入开启列表
heapq.heappush(open_list, (f, node))
return []
```
需要注意的是,这只是一个简单的实现,如果需要用于实际场景中的路径规划,还需要考虑更多的因素,比如地形、障碍物的形状、大小等。
阅读全文
相关推荐
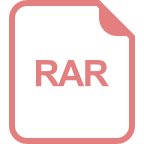
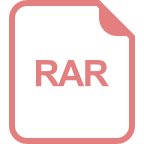
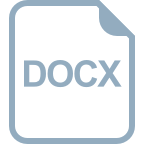
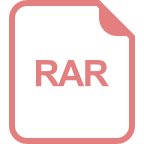
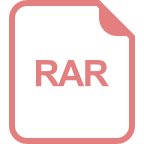
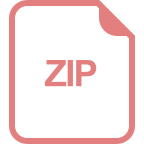
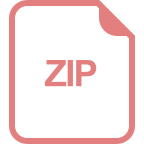
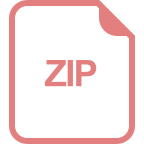
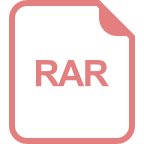
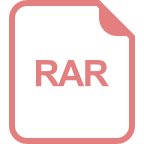
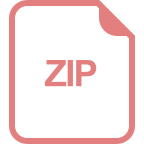
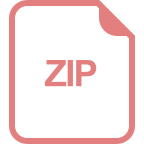
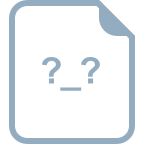
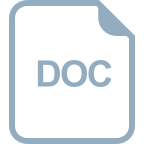