给定n种物品和一背包。物品i的重量是wi,其价值为vi,背包的容量为C。问应如何选择装入背包的物品,使得装入背包中物品的总价值最大? 用回溯算法求解该问题,找出问题的最优值和最优解。用C语言实现该算法。
时间: 2023-10-02 15:11:08 浏览: 23
这是一个经典的背包问题,可以使用回溯算法来解决。具体步骤如下:
1. 定义一个结构体来表示物品,包括重量、价值和是否被选中的标记。
```C
typedef struct Item {
int weight; // 物品重量
int value; // 物品价值
int selected; // 是否被选中
} Item;
```
2. 定义一个函数来计算当前状态下的总价值。
```C
int calculateValue(Item *items, int n) {
int value = 0;
for (int i = 0; i < n; i++) {
if (items[i].selected) {
value += items[i].value;
}
}
return value;
}
```
3. 定义一个回溯函数来搜索所有可能的状态,找到最优解。
```C
void backtrack(Item *items, int n, int capacity, int currentWeight, int currentValue, int *maxValue) {
if (currentWeight > capacity) {
return;
}
if (currentValue > *maxValue) {
*maxValue = currentValue;
}
for (int i = 0; i < n; i++) {
if (!items[i].selected) {
items[i].selected = 1;
backtrack(items, n, capacity, currentWeight + items[i].weight, currentValue + items[i].value, maxValue);
items[i].selected = 0;
}
}
}
```
4. 在主函数中定义物品数组,调用回溯函数,输出最优值和最优解。
```C
int main() {
int n = 5; // 物品数量
int capacity = 10; // 背包容量
Item items[5] = {{2, 6, 0}, {2, 3, 0}, {6, 5, 0}, {5, 4, 0}, {4, 6, 0}};
int maxValue = 0;
backtrack(items, n, capacity, 0, 0, &maxValue);
printf("最优值为:%d\n", maxValue);
printf("最优解为:");
for (int i = 0; i < n; i++) {
if (items[i].selected) {
printf("%d ", i);
}
}
printf("\n");
return 0;
}
```
注意,这里的物品数量、背包容量和物品的重量价值都是随意给定的,实际使用时需要根据具体情况进行调整。
阅读全文
相关推荐
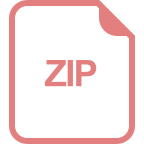
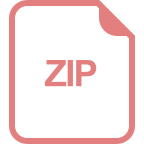
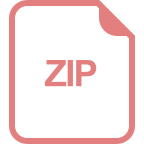








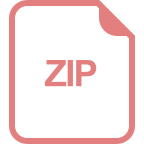
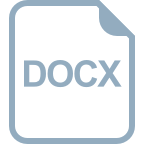
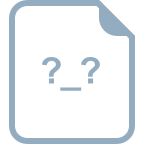
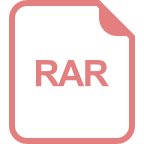
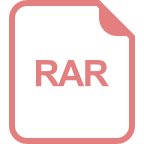
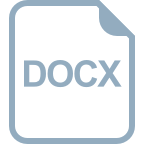