来个C语言贪吃蛇的代码
时间: 2023-08-31 09:23:12 浏览: 72
### 回答1:
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 20
#define HEIGHT 20
int score = ;
int speed = 200;
typedef struct _COORD {
int x;
int y;
} COORD;
typedef struct _SNAKE {
COORD head;
COORD body[WIDTH * HEIGHT];
int length;
int direction;
} SNAKE;
typedef struct _FOOD {
COORD pos;
int exist;
} FOOD;
void gotoxy(int x, int y) {
COORD pos = { x, y };
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), pos);
}
void init(SNAKE* snake, FOOD* food) {
snake->head.x = WIDTH / 2;
snake->head.y = HEIGHT / 2;
snake->body[].x = snake->head.x - 1;
snake->body[].y = snake->head.y;
snake->body[1].x = snake->head.x - 2;
snake->body[1].y = snake->head.y;
snake->length = 2;
snake->direction = 'd';
food->exist = ;
}
void draw(SNAKE* snake, FOOD* food) {
system("cls");
for (int i = ; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
for (int i = ; i < HEIGHT; i++) {
printf("#");
for (int j = ; j < WIDTH; j++) {
if (i == snake->head.y && j == snake->head.x) {
printf("O");
}
else if (i == food->pos.y && j == food->pos.x && food->exist) {
printf("F");
}
else {
int flag = ;
for (int k = ; k < snake->length; k++) {
if (i == snake->body[k].y && j == snake->body[k].x) {
printf("o");
flag = 1;
break;
}
}
if (!flag) {
printf(" ");
}
}
}
printf("#\n");
}
for (int i = ; i < WIDTH + 2; i++) {
printf("#");
}
printf("\n");
printf("Score: %d\n", score);
}
void update(SNAKE* snake, FOOD* food) {
COORD temp = snake->body[snake->length - 1];
for (int i = snake->length - 1; i > ; i--) {
snake->body[i] = snake->body[i - 1];
}
snake->body[] = snake->head;
snake->head = temp;
switch (snake->direction) {
case 'w':
snake->head.y--;
break;
case 'a':
snake->head.x--;
break;
case 's':
snake->head.y++;
break;
case 'd':
snake->head.x++;
break;
}
if (snake->head.x < || snake->head.x >= WIDTH || snake->head.y < || snake->head.y >= HEIGHT) {
printf("Game Over!\n");
exit();
}
for (int i = ; i < snake->length; i++) {
if (snake->head.x == snake->body[i].x && snake->head.y == snake->body[i].y) {
printf("Game Over!\n");
exit();
}
}
if (snake->head.x == food->pos.x && snake->head.y == food->pos.y) {
score += 10;
snake->length++;
if (speed > 50) {
speed -= 10;
}
food->exist = ;
}
}
void generate_food(FOOD* food) {
if (!food->exist) {
food->pos.x = rand() % WIDTH;
food->pos.y = rand() % HEIGHT;
food->exist = 1;
}
}
void input(SNAKE* snake) {
if (_kbhit()) {
char c = _getch();
switch (c) {
case 'w':
case 'a':
case 's':
case 'd':
if (abs(snake->direction - c) != 2) {
snake->direction = c;
}
break;
}
}
}
int main() {
srand((unsigned)time(NULL));
SNAKE snake;
FOOD food;
init(&snake, &food);
while (1) {
draw(&snake, &food);
update(&snake, &food);
generate_food(&food);
input(&snake);
Sleep(speed);
}
return ;
}
### 回答2:
以下是一个基于 C 语言的简单贪吃蛇游戏的代码:
```c
#include <stdio.h>
#include <conio.h>
#include <windows.h>
#define WIDTH 20 // 游戏界面的宽度
#define HEIGHT 20 // 游戏界面的高度
int score = 0; // 当前得分
int gameOver = 0; // 游戏是否结束
int snakeX, snakeY; // 蛇头的坐标
int fruitX, fruitY; // 水果的坐标
int tailX[100], tailY[100]; // 蛇身的坐标数组
int nTail = 0; // 当前蛇身的长度
enum Direction { STOP = 0, LEFT, RIGHT, UP, DOWN }; // 蛇的移动方向
enum Direction dir;
void Setup()
{
gameOver = 0;
dir = STOP;
// 设置初始位置
snakeX = WIDTH / 2;
snakeY = HEIGHT / 2;
// 在随机位置生成水果
srand(time(NULL));
fruitX = rand() % WIDTH;
fruitY = rand() % HEIGHT;
score = 0;
}
void Draw()
{
system("cls"); // 清空控制台
// 打印上边界
for (int i = 0; i < WIDTH + 2; i++)
printf("#");
printf("\n");
// 打印每一行
for (int i = 0; i < HEIGHT; i++)
{
for (int j = 0; j < WIDTH; j++)
{
// 打印左边界、蛇头、蛇身、水果等
if (j == 0)
printf("#");
if (i == snakeY && j == snakeX)
printf("O");
else if (i == fruitY && j == fruitX)
printf("F");
else
{
int isTail = 0;
for (int k = 0; k < nTail; k++)
{
// 打印蛇身
if (tailX[k] == j && tailY[k] == i)
{
printf("o");
isTail = 1;
}
}
if (!isTail)
printf(" ");
}
// 打印右边界
if (j == WIDTH - 1)
printf("#");
}
printf("\n");
}
// 打印下边界
for (int i = 0; i < WIDTH + 2; i++)
printf("#");
printf("\n");
// 打印得分
printf("Score: %d\n", score);
}
void Input()
{
// 判断按键
if (_kbhit())
{
switch (_getch())
{
case 'a':
dir = LEFT;
break;
case 'd':
dir = RIGHT;
break;
case 'w':
dir = UP;
break;
case 's':
dir = DOWN;
break;
case 'x':
gameOver = 1;
break;
}
}
}
void Logic()
{
// 更新蛇身的坐标
int prevX = tailX[0];
int prevY = tailY[0];
int prev2X, prev2Y;
tailX[0] = snakeX;
tailY[0] = snakeY;
for (int i = 1; i < nTail; i++)
{
prev2X = tailX[i];
prev2Y = tailY[i];
tailX[i] = prevX;
tailY[i] = prevY;
prevX = prev2X;
prevY = prev2Y;
}
// 更新蛇头的坐标
switch (dir)
{
case LEFT:
snakeX--;
break;
case RIGHT:
snakeX++;
break;
case UP:
snakeY--;
break;
case DOWN:
snakeY++;
break;
}
// 检查蛇是否碰到边界或自己的身体
if (snakeX < 0 || snakeX >= WIDTH || snakeY < 0 || snakeY >= HEIGHT)
gameOver = 1;
for (int i = 0; i < nTail; i++)
if (tailX[i] == snakeX && tailY[i] == snakeY)
gameOver = 1;
// 检查是否吃到水果
if (snakeX == fruitX && snakeY == fruitY)
{
score += 10;
fruitX = rand() % WIDTH;
fruitY = rand() % HEIGHT;
nTail++;
}
}
int main()
{
Setup();
while (!gameOver)
{
Draw();
Input();
Logic();
Sleep(10); // 控制游戏速度
}
return 0;
}
```
这段代码实现了一个简单的贪吃蛇游戏。游戏界面为一个矩形框,蛇头用大写字母 "O" 表示,蛇身用小写字母 "o" 表示,水果用字母 "F" 表示。通过键盘的方向键控制蛇的移动方向,游戏结束条件为蛇碰到边界或自己的身体。吃到水果会得分,并在随机位置生成新的水果。游戏使用 Windows.h 头文件中的 Sleep 函数控制游戏速度。
### 回答3:
下面是一个简单的C语言贪吃蛇的代码示例:
```c
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <unistd.h>
#include <time.h>
#define WIDTH 20
#define HEIGHT 20
#define UP 'w'
#define DOWN 's'
#define LEFT 'a'
#define RIGHT 'd'
#define INIT_LENGTH 3
typedef struct
{
int x;
int y;
} Coordinate;
Coordinate body[WIDTH*HEIGHT];
Coordinate food;
int length = INIT_LENGTH;
char dir = RIGHT;
void drawBoard()
{
system("cls");
for (int i = 0; i < HEIGHT; i++) {
for (int j = 0; j < WIDTH; j++) {
if (i == 0 || i == HEIGHT-1 || j == 0 || j == WIDTH-1) {
printf("*");
} else if (i == food.y && j == food.x) {
printf("@");
} else {
int isBodyPart = 0;
for (int k = 0; k < length; k++) {
if (body[k].x == j && body[k].y == i) {
printf("O");
isBodyPart = 1;
break;
}
}
if (!isBodyPart) {
printf(" ");
}
}
}
printf("\n");
}
}
void generateFood()
{
srand(time(NULL));
while (1) {
int x = rand() % (WIDTH - 2) + 1;
int y = rand() % (HEIGHT - 2) + 1;
int isOnBody = 0;
for (int i = 0; i < length; i++) {
if (x == body[i].x && y == body[i].y) {
isOnBody = 1;
break;
}
}
if (!isOnBody) {
food.x = x;
food.y = y;
break;
}
}
}
int isGameOver()
{
if (body[0].x <= 0 || body[0].x >= WIDTH-1 || body[0].y <= 0 || body[0].y >= HEIGHT-1) {
return 1;
}
for (int i = 1; i < length; i++) {
if (body[0].x == body[i].x && body[0].y == body[i].y) {
return 1;
}
}
return 0;
}
void move()
{
for (int i = length-1; i > 0; i--) {
body[i].x = body[i-1].x;
body[i].y = body[i-1].y;
}
switch (dir) {
case UP:
body[0].y--;
break;
case DOWN:
body[0].y++;
break;
case LEFT:
body[0].x--;
break;
case RIGHT:
body[0].x++;
break;
}
if (body[0].x == food.x && body[0].y == food.y) {
length++;
generateFood();
}
}
int main()
{
generateFood();
while (!isGameOver()) {
if (_kbhit()) {
dir = _getch();
}
move();
drawBoard();
usleep(300000);
}
printf("Game Over!");
return 0;
}
```
该代码使用了Windows特定的函数 `_kbhit()` 和 `_getch()` 来监听键盘输入,因此需要在Windows环境下编译运行。
相关推荐
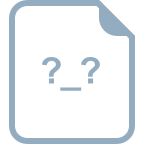













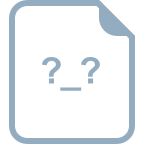