给出蜂群算法解决Tsp问题的实例代码
时间: 2023-11-01 08:07:55 浏览: 54
以下是一个使用蜂群算法解决TSP问题的Python代码示例:
```python
import numpy as np
import random
# 定义TSP问题的距离矩阵
distance_matrix = np.array([
[0, 10, 15, 20],
[10, 0, 35, 25],
[15, 35, 0, 30],
[20, 25, 30, 0]
])
# 蚂蚁类
class Ant:
def __init__(self, start_city, alpha, beta, pheromone_matrix):
self.start_city = start_city # 起始城市
self.alpha = alpha # alpha参数
self.beta = beta # beta参数
self.pheromone_matrix = pheromone_matrix # 信息素矩阵
self.visited = [start_city] # 已访问城市列表
self.unvisited = set(range(len(distance_matrix))) - set([start_city]) # 未访问城市列表
# 计算从当前城市到所有未访问城市的转移概率
def compute_probabilities(self):
probabilities = []
total = 0
for city in self.unvisited:
pheromone = self.pheromone_matrix[self.visited[-1]][city]
distance = distance_matrix[self.visited[-1]][city]
probability = pow(pheromone, self.alpha) * pow(1.0/distance, self.beta)
probabilities.append((city, probability))
total += probability
# 归一化
probabilities = [(city, probability/total) for (city, probability) in probabilities]
return probabilities
# 选择下一个城市
def choose_next_city(self):
probabilities = self.compute_probabilities()
r = random.uniform(0, 1)
total = 0
for (city, probability) in probabilities:
total += probability
if total >= r:
return city
# 蚂蚁走一步
def move(self):
next_city = self.choose_next_city()
self.visited.append(next_city)
self.unvisited.remove(next_city)
# 计算路径长度
def path_length(self):
length = 0
for i in range(len(self.visited)-1):
length += distance_matrix[self.visited[i]][self.visited[i+1]]
length += distance_matrix[self.visited[-1]][self.visited[0]]
return length
# 蜂群算法类
class BeeColony:
def __init__(self, num_ants, num_iterations, alpha, beta, rho, q):
self.num_ants = num_ants # 蚂蚁数量
self.num_iterations = num_iterations # 迭代次数
self.alpha = alpha # alpha参数
self.beta = beta # beta参数
self.rho = rho # 信息素挥发因子
self.q = q # 信息素增加强度
self.pheromone_matrix = np.ones((len(distance_matrix), len(distance_matrix))) # 信息素矩阵
self.best_path = None # 最佳路径
self.best_path_length = np.inf # 最佳路径长度
# 运行蜂群算法
def run(self):
for iteration in range(self.num_iterations):
# 初始化蚂蚁
ants = [Ant(i, self.alpha, self.beta, self.pheromone_matrix) for i in range(self.num_ants)]
# 蚂蚁走一步
for ant in ants:
while ant.unvisited:
ant.move()
# 更新最佳路径
if ant.path_length() < self.best_path_length:
self.best_path = ant.visited
self.best_path_length = ant.path_length()
# 更新信息素
self.update_pheromone(ants)
# 输出当前迭代的结果
print("Iteration", iteration+1, "Best Path", self.best_path, "Best Path Length", self.best_path_length)
# 更新信息素
def update_pheromone(self, ants):
# 信息素挥发
self.pheromone_matrix = (1-self.rho) * self.pheromone_matrix
# 信息素增加
for ant in ants:
path_length = ant.path_length()
for i in range(len(ant.visited)-1):
city1 = ant.visited[i]
city2 = ant.visited[i+1]
self.pheromone_matrix[city1][city2] += self.q/path_length
self.pheromone_matrix[city2][city1] += self.q/path_length
# 运行蜂群算法
bee_colony = BeeColony(num_ants=10, num_iterations=100, alpha=1, beta=5, rho=0.5, q=100)
bee_colony.run()
```
相关推荐
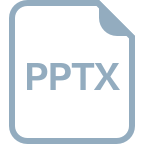
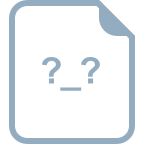














