给我一个人工蜂群算法解决tsp代码
时间: 2023-08-06 18:16:49 浏览: 67
以下是一个简单的Python代码实现,使用人工蜂群算法解决TSP问题:
```python
import numpy as np
import random
import math
# 初始化参数
num_city = 20 # 城市个数
num_bee = 20 # 蜜蜂个数
max_iter = 100 # 最大迭代次数
limit = 10 # 每只蜜蜂最大搜索次数
# 初始化城市坐标
city_coords = np.random.rand(num_city, 2) * 100
# 计算城市之间的距离矩阵
def calc_distance_matrix(coords):
dist_matrix = np.zeros((num_city, num_city))
for i in range(num_city):
for j in range(num_city):
dist_matrix[i][j] = math.sqrt((coords[i][0]-coords[j][0])**2 + (coords[i][1]-coords[j][1])**2)
return dist_matrix
dist_matrix = calc_distance_matrix(city_coords)
# 初始化蜜蜂群体
class Bee:
def __init__(self):
self.path = np.random.permutation(num_city) # 随机初始化路径
self.dist = self.calc_path_distance() # 计算路径距离
self.counter = 0 # 搜索次数计数器
# 计算路径距离
def calc_path_distance(self):
total_dist = 0
for i in range(num_city-1):
total_dist += dist_matrix[self.path[i]][self.path[i+1]]
total_dist += dist_matrix[self.path[num_city-1]][self.path[0]]
return total_dist
# 搜索新的路径
def search_new_path(self):
while True:
new_path = self.path.copy()
i, j = np.random.choice(num_city, 2, replace=False)
new_path[i], new_path[j] = new_path[j], new_path[i]
new_dist = self.calc_path_distance()
if new_dist < self.dist:
self.path = new_path
self.dist = new_dist
self.counter = 0
break
else:
self.counter += 1
if self.counter >= limit:
break
bees = [Bee() for i in range(num_bee)]
# 迭代搜索
for iter in range(max_iter):
for bee in bees:
bee.search_new_path()
# 根据路径距离排序,选择最优蜜蜂
bees.sort(key=lambda bee: bee.dist)
best_bee = bees[0]
# 生成新的蜜蜂群体
new_bees = []
for i in range(num_bee):
new_bee = Bee()
j = random.randint(0, int(num_bee/2))
new_bee.path = bees[j].path.copy()
new_bee.search_new_path()
new_bees.append(new_bee)
bees = new_bees
print("Iteration %d, Best Distance: %.2f" % (iter+1, best_bee.dist))
# 输出最优路径
print("Best Path: ", best_bee.path)
```
这段代码中,首先初始化城市坐标和距离矩阵。然后定义了一个Bee类,表示一只蜜蜂,包括当前路径、路径长度和搜索次数计数器等属性,以及计算路径长度和搜索新路径的方法。接着初始化蜜蜂群体,包括多只蜜蜂。在每次迭代中,每只蜜蜂搜索新的路径,更新自己的路径和路径长度,并根据路径长度排序选择最优蜜蜂。然后生成新的蜜蜂群体,包括多只新的蜜蜂,其中一部分蜜蜂的路径参考当前最优蜜蜂的路径。最后输出最优路径。
相关推荐
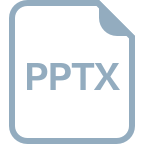
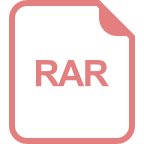
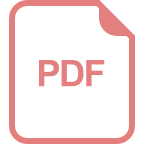














