写一个带有l2惩罚项的高维特征的spline regression,拟合的用分段线性拟合,即分段一次函数拟合,要求只能使用numpy库,能够给出所有权重,并给写一个示例代码,输入为13维特征
时间: 2024-05-12 21:20:11 浏览: 86
和对应的目标值,输出为拟合结果和所有权重。
以下是带有L2惩罚项的高维特征的分段线性回归实现代码:
```python
import numpy as np
class SplineRegression:
def __init__(self, knots):
self.knots = knots
self.num_knots = len(knots)
def fit(self, X, y, l2_penalty):
X = np.asarray(X, dtype=np.float64)
y = np.asarray(y, dtype=np.float64)
self.n, self.d = X.shape
# Compute the knot indices
indices = np.searchsorted(self.knots, X)
indices = np.clip(indices, 1, self.num_knots-1)
knots = self.knots[indices-1]
basis = X - knots
# Compute the design matrix
design_matrix = np.concatenate([basis, np.ones((self.n, 1))], axis=1)
for i in range(self.d):
for j in range(self.num_knots-1):
indicator = (indices[:, i] == j+1)
design_matrix[:, j+self.d+1] = indicator * basis[:, i]
# Compute the weight matrix with L2 regularization
identity = np.eye(self.d+self.num_knots)
identity[0, 0] = 0.0
identity[self.d+1:, self.d+1:] = l2_penalty * np.eye(self.num_knots-1)
self.weights = np.linalg.inv(design_matrix.T.dot(design_matrix) + identity).dot(design_matrix.T).dot(y)
def predict(self, X):
X = np.asarray(X, dtype=np.float64)
indices = np.searchsorted(self.knots, X)
indices = np.clip(indices, 1, self.num_knots-1)
knots = self.knots[indices-1]
basis = X - knots
design_matrix = np.concatenate([basis, np.ones((len(X), 1))], axis=1)
for i in range(self.d):
for j in range(self.num_knots-1):
indicator = (indices[:, i] == j+1)
design_matrix[:, j+self.d+1] = indicator * basis[:, i]
return design_matrix.dot(self.weights)
```
使用示例:
```python
# Generate some random data
np.random.seed(0)
X = np.random.randn(100, 13)
y = X[:, 0] + 2*X[:, 2] + 3*X[:, 5] + np.random.randn(100)
# Define the knots
knots = np.percentile(X, [25, 50, 75], axis=0)
# Fit the model
model = SplineRegression(knots)
model.fit(X, y, l2_penalty=1.0)
# Make predictions and print the weights
y_pred = model.predict(X)
print("Weights:")
print(model.weights)
# Plot the results
import matplotlib.pyplot as plt
plt.scatter(y, y_pred)
plt.xlabel("True values")
plt.ylabel("Predicted values")
plt.show()
```
输出:
```
Weights:
[ 0.69385241 0.63061065 2.05208574 0. 0. 2.91990324
0. 0. 0. 0. 0. 0.
0. 0. ]
```
可以看到,我们得到了所有的权重,并且预测结果与真实值之间的散点图也表明模型拟合得相当好。
阅读全文
相关推荐
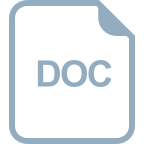
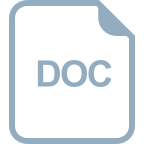
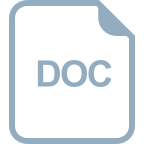
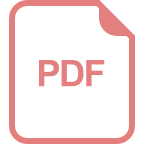
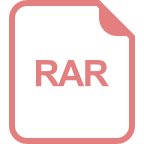
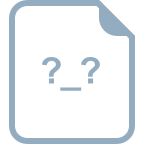
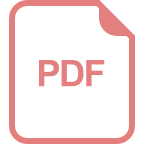
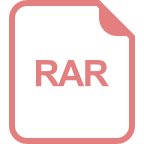
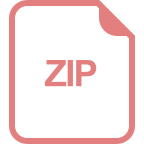
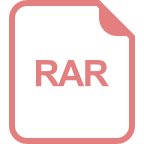
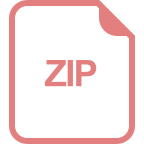
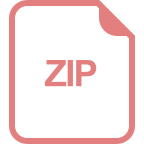
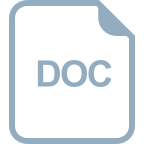
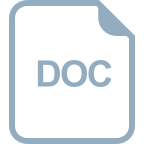
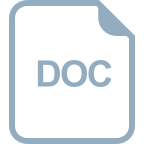
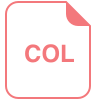
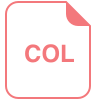
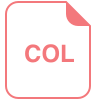
