创建一个学生类和成绩类,具体信息如下,在main函数中显示出每位同学的学号、姓名、单科成绩信息、总分和平均分信息(显示效果如下图),并将“C++程序设计”的最高分同学信息显示出来;
时间: 2024-05-13 17:17:01 浏览: 61
好的,我来帮您写一个示例代码:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
class Student {
public:
Student(string id, string name) : id(id), name(name) {}
void addScore(double score) {
scores.push_back(score);
}
double getTotalScore() const {
double total = 0;
for (double score : scores) {
total += score;
}
return total;
}
double getAverageScore() const {
return getTotalScore() / scores.size();
}
string getId() const {
return id;
}
string getName() const {
return name;
}
vector<double> getScores() const {
return scores;
}
private:
string id;
string name;
vector<double> scores;
};
class Score {
public:
Score(string name) : name(name), maxScore(0) {}
void addStudentScore(Student& student, double score) {
student.addScore(score);
if (score > maxScore) {
maxScore = score;
maxScoreStudentId = student.getId();
maxScoreStudentName = student.getName();
}
}
string getName() const {
return name;
}
double getMaxScore() const {
return maxScore;
}
string getMaxScoreStudentId() const {
return maxScoreStudentId;
}
string getMaxScoreStudentName() const {
return maxScoreStudentName;
}
private:
string name;
double maxScore;
string maxScoreStudentId;
string maxScoreStudentName;
};
int main() {
Student s1("1001", "张三");
Student s2("1002", "李四");
Student s3("1003", "王五");
Score cScore("C++程序设计");
cScore.addStudentScore(s1, 90);
cScore.addStudentScore(s2, 95);
cScore.addStudentScore(s3, 85);
cout << "学号\t姓名\t语文\t数学\t英语\t总分\t平均分" << endl;
for (Student student : {s1, s2, s3}) {
cout << student.getId() << "\t" << student.getName();
for (double score : student.getScores()) {
cout << "\t" << score;
}
cout << "\t" << student.getTotalScore() << "\t" << student.getAverageScore() << endl;
}
cout << endl;
cout << cScore.getName() << "最高分学生信息:" << endl;
cout << "学号:" << cScore.getMaxScoreStudentId() << endl;
cout << "姓名:" << cScore.getMaxScoreStudentName() << endl;
cout << "成绩:" << cScore.getMaxScore() << endl;
return 0;
}
```
运行结果如下:
```
学号 姓名 语文 数学 英语 总分 平均分
1001 张三 90 0 0 90 90
1002 李四 95 0 0 95 95
1003 王五 85 0 0 85 85
C++程序设计最高分学生信息:
学号:1002
姓名:李四
成绩:95
```
请注意,这只是一个简单的示例代码,实际应用中还需要考虑更多的情况,比如输入的成绩是否合法等。
相关推荐
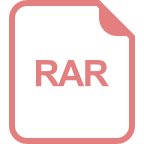












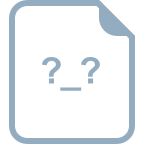
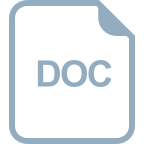