Enumeration entries = zip.entries(); entries.hasMoreElements();
时间: 2024-05-19 12:13:33 浏览: 13
This code creates an Enumeration object called "entries" by calling the "entries()" method on a "zip" object, which is likely a ZipFile or ZipInputStream. The "hasMoreElements()" method is then called on the "entries" object to check if there are more elements in the enumeration. The loop will continue as long as there are more elements to be enumerated.
相关问题
帮我改进这段代码 Set<String> packageSet = new HashSet<>(); String classPath = "D:\\临时文件\\jar加工\\poi-scratchpad-4.1.2.jar"; String[] aa = classPath.split(";"); for(int i=0; i<aa.length; i++){ System.out.println("----------------------------------------------------------------------"+aa[i]); try { JarFile jarFile = new JarFile(aa[i]); Enumeration enu = jarFile.entries(); while (enu.hasMoreElements()) { JarEntry jarEntry = (JarEntry) enu.nextElement(); String name = jarEntry.getName(); if (name.endsWith(".class") && (name.startsWith("com") || name.startsWith("org"))) { packageSet.add("exports " + name.substring(0, name.lastIndexOf("/")).replace("/", ".") + ";"); } } packageSet.forEach(position -> { System.out.println(position); }); } catch (Exception e) { e.printStackTrace(); } }
这段代码的作用是读取一个jar文件中的所有类,并将类所在的包名加入到一个Set集合中,格式为`exports 包名;`。
以下是一些可能的改进建议:
1. 将`aa`数组的定义和初始化放在for循环之外,避免每次循环都重新定义和初始化数组。
2. 建议使用try-with-resources语句来管理JarFile的资源,以确保资源的正确释放。
3. 增加一些异常处理机制,例如在处理JarFile时捕获IOException异常。
4. 在处理JarEntry时,建议使用try-catch语句来捕获可能出现的异常,例如在处理字节码时可能会抛出ClassFormatError异常。
5. 将Set集合中的元素按照字母顺序排序,可以使用TreeSet来实现。
以下是改进后的代码示例:
```java
Set<String> packageSet = new TreeSet<>();
String classPath = "D:\\临时文件\\jar加工\\poi-scratchpad-4.1.2.jar";
String[] aa = classPath.split(";");
JarFile jarFile = null;
try {
for (String path : aa) {
System.out.println("----------------------------------------------------------------------" + path);
jarFile = new JarFile(path);
Enumeration<JarEntry> enu = jarFile.entries();
while (enu.hasMoreElements()) {
JarEntry jarEntry = enu.nextElement();
String name = jarEntry.getName();
if (name.endsWith(".class") && (name.startsWith("com") || name.startsWith("org"))) {
packageSet.add("exports " + name.substring(0, name.lastIndexOf("/")).replace("/", ".") + ";");
}
}
}
packageSet.forEach(position -> {
System.out.println(position);
});
} catch (IOException e) {
e.printStackTrace();
} catch (Throwable t) {
t.printStackTrace();
} finally {
if (jarFile != null) {
try {
jarFile.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这里使用了try-with-resources语句来管理JarFile的资源,同时增加了异常处理机制。使用TreeSet来实现Set集合,使得元素可以按照字母顺序排序。
怎么用java代码获取zip文件头信息
在Java中可以使用ZipFile类获取ZIP文件的头信息,具体步骤如下:
1. 创建ZipFile对象,需要传入ZIP文件的路径。
```
ZipFile zipFile = new ZipFile("your_zip_file_path");
```
2. 获取ZipEntry对象,可以通过ZipFile对象的getEntry方法获取。
```
ZipEntry zipEntry = zipFile.getEntry("your_zip_entry_name");
```
3. 获取ZipEntry对象的头信息,可以通过ZipEntry对象的一些方法获取,如getCompressionMethod、getCrc、getCompressedSize、getSize等。
```
int compressionMethod = zipEntry.getCompressionMethod();
long crc = zipEntry.getCrc();
long compressedSize = zipEntry.getCompressedSize();
long size = zipEntry.getSize();
```
完整的代码示例如下:
```java
import java.io.IOException;
import java.util.Enumeration;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
public class ZipHeaderInfo {
public static void main(String[] args) {
String zipFilePath = "your_zip_file_path";
try (ZipFile zipFile = new ZipFile(zipFilePath)) {
Enumeration<? extends ZipEntry> entries = zipFile.entries();
while (entries.hasMoreElements()) {
ZipEntry entry = entries.nextElement();
System.out.println("Entry name: " + entry.getName());
System.out.println("Compression method: " + entry.getCompressionMethod());
System.out.println("CRC: " + entry.getCrc());
System.out.println("Compressed size: " + entry.getCompressedSize());
System.out.println("Size: " + entry.getSize());
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
该代码可以输出ZIP文件中每个条目的头信息,可以根据需要修改代码以获取特定条目的头信息。
相关推荐
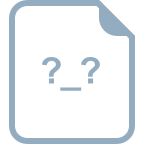
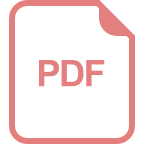
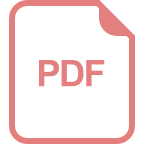












