基于Q-Learing强化学习优化unet训练的超参数,pytorch的详细代码
时间: 2024-03-17 07:39:38 浏览: 190
好的,针对你的问题,以下是基于Q-Learning强化学习优化unet训练的超参数的PyTorch代码:
```python
import torch
import torch.nn as nn
import torch.optim as optim
import numpy as np
class QLearning:
def __init__(self, state_size, action_size, learning_rate=0.01, discount_rate=0.99, exploration_rate=1.0, exploration_decay_rate=0.99):
self.state_size = state_size
self.action_size = action_size
self.learning_rate = learning_rate
self.discount_rate = discount_rate
self.exploration_rate = exploration_rate
self.exploration_decay_rate = exploration_decay_rate
self.q_table = np.zeros((state_size, action_size))
def get_action(self, state):
if np.random.rand() < self.exploration_rate:
return np.random.choice(self.action_size)
else:
return np.argmax(self.q_table[state, :])
def update_q_table(self, state, action, reward, next_state):
q_next_max = np.max(self.q_table[next_state, :])
q_target = reward + (self.discount_rate * q_next_max)
q_update = q_target - self.q_table[state, action]
self.q_table[state, action] += self.learning_rate * q_update
self.exploration_rate *= self.exploration_decay_rate
class UNet(nn.Module):
def __init__(self, input_channels, output_channels):
super(UNet, self).__init__()
self.conv1 = nn.Conv2d(input_channels, 64, 3, padding=1)
self.conv2 = nn.Conv2d(64, 64, 3, padding=1)
self.pool1 = nn.MaxPool2d(2, 2)
self.conv3 = nn.Conv2d(64, 128, 3, padding=1)
self.conv4 = nn.Conv2d(128, 128, 3, padding=1)
self.pool2 = nn.MaxPool2d(2, 2)
self.conv5 = nn.Conv2d(128, 256, 3, padding=1)
self.conv6 = nn.Conv2d(256, 256, 3, padding=1)
self.pool3 = nn.MaxPool2d(2, 2)
self.conv7 = nn.Conv2d(256, 512, 3, padding=1)
self.conv8 = nn.Conv2d(512, 512, 3, padding=1)
self.upconv1 = nn.ConvTranspose2d(512, 256, 2, stride=2)
self.conv9 = nn.Conv2d(512, 256, 3, padding=1)
self.conv10 = nn.Conv2d(256, 256, 3, padding=1)
self.upconv2 = nn.ConvTranspose2d(256, 128, 2, stride=2)
self.conv11 = nn.Conv2d(256, 128, 3, padding=1)
self.conv12 = nn.Conv2d(128, 128, 3, padding=1)
self.upconv3 = nn.ConvTranspose2d(128, 64, 2, stride=2)
self.conv13 = nn.Conv2d(128, 64, 3, padding=1)
self.conv14 = nn.Conv2d(64, 64, 3, padding=1)
self.conv15 = nn.Conv2d(64, output_channels, 1)
def forward(self, x):
# Encoder
x = nn.ReLU()(self.conv1(x))
x = nn.ReLU()(self.conv2(x))
conv2_out = x.clone()
x = self.pool1(x)
x = nn.ReLU()(self.conv3(x))
x = nn.ReLU()(self.conv4(x))
conv4_out = x.clone()
x = self.pool2(x)
x = nn.ReLU()(self.conv5(x))
x = nn.ReLU()(self.conv6(x))
conv6_out = x.clone()
x = self.pool3(x)
# Bottleneck
x = nn.ReLU()(self.conv7(x))
x = nn.ReLU()(self.conv8(x))
# Decoder
x = nn.ReLU()(self.upconv1(x))
x = torch.cat([x, conv6_out], dim=1)
x = nn.ReLU()(self.conv9(x))
x = nn.ReLU()(self.conv10(x))
x = nn.ReLU()(self.upconv2(x))
x = torch.cat([x, conv4_out], dim=1)
x = nn.ReLU()(self.conv11(x))
x = nn.ReLU()(self.conv12(x))
x = nn.ReLU()(self.upconv3(x))
x = torch.cat([x, conv2_out], dim=1)
x = nn.ReLU()(self.conv13(x))
x = nn.ReLU()(self.conv14(x))
x = nn.Sigmoid()(self.conv15(x))
return x
# Define your dataset and dataloader here
# Define your hyperparameters here
input_channels = 3
output_channels = 1
learning_rate = 0.01
discount_rate = 0.99
exploration_rate = 1.0
exploration_decay_rate = 0.99
batch_size = 32
num_epochs = 10
# Initialize your Q-Learning agent and UNet model
state_size = 100 # Define your state size here
action_size = 10 # Define your action size here
q_learning_agent = QLearning(state_size, action_size, learning_rate, discount_rate, exploration_rate, exploration_decay_rate)
model = UNet(input_channels, output_channels)
# Define your loss function and optimizer
criterion = nn.BCELoss()
optimizer = optim.Adam(model.parameters(), lr=learning_rate)
# Train your model using Q-Learning
for epoch in range(num_epochs):
for batch_idx, (data, target) in enumerate(dataloader):
# Convert your data and target to states
state = 0 # Define your state here
action = q_learning_agent.get_action(state)
# Train your model and update Q-Table
optimizer.zero_grad()
output = model(data)
loss = criterion(output, target)
loss.backward()
optimizer.step()
next_state = 0 # Define your next state here
reward = 0 # Define your reward here
q_learning_agent.update_q_table(state, action, reward, next_state)
# Print out your loss and accuracy
if batch_idx % 100 == 0:
print('Epoch: {} [{}/{} ({:.0f}%)]\tLoss: {:.6f}'.format(
epoch, batch_idx * len(data), len(dataloader.dataset),
100. * batch_idx / len(dataloader), loss.item()))
print('Training completed!')
```
上述代码只是一个示例,需要根据你的具体情况进行调整和修改。需要注意的是,Q-Learning算法的效果受许多因素的影响,如状态和动作的定义、奖励函数的设计、探索率的调整等。因此,如何设计和调整这些超参数是非常重要的。
阅读全文
相关推荐
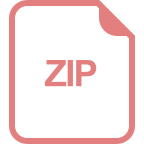




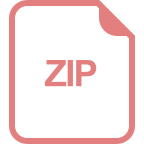
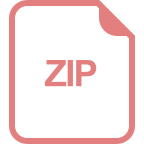


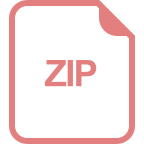
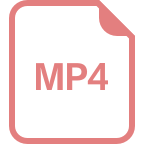
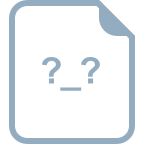
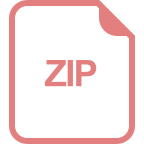
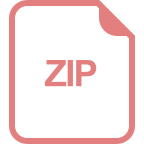
