Select high-confidence predictions代码怎么写
时间: 2024-02-28 11:55:58 浏览: 21
这个问题需要具体指明是在哪个领域或者是使用哪种算法进行预测。但我可以给出一个通用的思路。
对于任何一种预测算法,可以通过给定一个阈值,将预测结果中置信度高于这个阈值的结果筛选出来,作为高置信度的预测结果。比如在机器学习中,可以使用分类器的预测概率来作为置信度,设定一个概率阈值,将概率大于该阈值的预测结果保留下来。在深度学习中,可以使用神经网络的softmax输出结果作为置信度,类似地设定一个阈值,将输出结果中置信度高于该阈值的结果筛选出来。
下面是一个示例的伪代码:
```
def select_high_confidence_predictions(predictions, threshold):
high_confidence_predictions = []
for prediction in predictions:
confidence = get_confidence(prediction) # 获取预测结果的置信度
if confidence >= threshold:
high_confidence_predictions.append(prediction)
return high_confidence_predictions
```
在具体实现中,需要根据不同的预测算法和模型结构,编写对应的获取置信度的函数。
相关问题
xu-white模型代码
Xu-White模型是一种用于时间序列数据估计的统计模型。它基于传统的ARIMA模型,通过引入外生因素的影响来提高预测准确度。
下面是Xu-White模型的部分代码:
1. 数据预处理:首先,需要导入所需的Python库和数据集。在这个模型中,我们通常使用pandas库加载和处理数据。然后,我们将数据集拆分为训练集和测试集。
```python
import pandas as pd
# 导入数据
data = pd.read_csv('data.csv')
# 拆分数据集
train_data = data[:200]
test_data = data[200:]
```
2. 定义模型:接下来,我们需要定义Xu-White模型。该模型的基本思想是将ARIMA模型的误差项分解为两个部分:内生误差和外生误差。其中,内生误差由ARIMA模型自身所能解释,外生误差则由外部因素决定。
```python
import statsmodels.api as sm
# 定义模型
model = sm.XuWhite(y=train_data, x=train_data_exog, order=(3, 1, 0))
```
3. 模型训练和拟合:接下来,我们使用模型来训练和拟合数据。通过估计模型参数,我们可以得到最佳拟合。
```python
# 模型训练
model_fit = model.fit()
# 打印参数估计结果
print(model_fit.params)
```
4. 模型预测:训练好模型后,我们可以使用模型来进行预测。这里我们使用训练好的模型对测试集进行预测,并计算预测准确度。
```python
# 模型预测
predictions = model_fit.predict(start=len(train_data), end=len(train_data)+len(test_data)-1)
# 计算预测准确度
mse = mean_squared_error(test_data['target'], predictions)
```
这只是Xu-White模型的部分代码示例,实际应用中还需要进行更多的数据处理、模型优化和结果评估。
DeepLabCut-live实例代码
DeepLabCut-live是一个基于DeepLabCut的实时姿态估计工具。以下是一个示例代码,展示如何使用DeepLabCut-live来实时估计姿态。
```python
import cv2
import numpy as np
import deeplabcut_live
# Load the config file and the trained model
config_file = 'path/to/config.yaml'
dlc_model = 'path/to/trained/model.h5'
# Define the video capture device
cap = cv2.VideoCapture(0)
# Set up the DeepLabCut-live object
dlc = deeplabcut_live.DLCModel(config_file, dlc_model)
while True:
# Capture frame-by-frame
ret, frame = cap.read()
# Flip the frame horizontally for intuitive movement
frame = cv2.flip(frame, 1)
# Process the frame with DeepLabCut-live
dlc.process_frame(frame)
# Draw the predicted landmarks on the frame
frame = dlc.draw_predictions(frame)
# Display the resulting frame
cv2.imshow('frame', frame)
# Exit if 'q' is pressed
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# Release the capture
cap.release()
cv2.destroyAllWindows()
```
在上述代码中,我们首先导入了所需的库和DeepLabCut-live模块。然后,我们加载了配置文件和训练好的模型。接着,我们定义了视频捕获设备并初始化了DeepLabCut-live对象。然后,我们开始循环读取帧并对每一帧进行姿态估计和绘制。最后,我们展示了结果,并在按下'q'键时退出循环。
请注意,在使用此代码之前,需要安装DeepLabCut和DeepLabCut-live,并创建一个配置文件和训练模型。
相关推荐
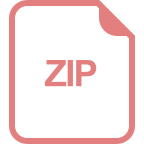












