基于c语言的六子棋Alpha-Beta剪枝算法来搜索博弈树
时间: 2024-06-12 21:06:05 浏览: 19
六子棋是一种类似于五子棋的棋类游戏,玩家需要在棋盘上落子,先将六个棋子连成一条线即可获胜。Alpha-Beta剪枝算法是一种常用于博弈树搜索的算法,可以有效地减少搜索的时间和空间复杂度。
以下是基于c语言的六子棋Alpha-Beta剪枝算法来搜索博弈树的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define BOARD_SIZE 15
#define MAX_DEPTH 4
int board[BOARD_SIZE][BOARD_SIZE]; // 棋盘
int depth; // 搜索深度
int evaluate(int player) // 评估函数,估算当前局面的价值
{
int score = 0;
// TODO: 实现评估函数
return score;
}
bool is_win(int player) // 判断是否获胜
{
// TODO: 实现判断获胜的函数
return false;
}
int alpha_beta(int player, int alpha, int beta, int depth) // Alpha-Beta剪枝搜索算法
{
if (depth == 0 || is_win(player)) // 达到搜索深度或者获胜,返回估值
{
return evaluate(player);
}
int best_score = player == 1 ? -9999 : 9999; // 初始化最佳分数
for (int i = 0; i < BOARD_SIZE; i++)
{
for (int j = 0; j < BOARD_SIZE; j++)
{
if (board[i][j] != 0) // 如果该位置已经有棋子,跳过
{
continue;
}
board[i][j] = player; // 在该位置落子
int score = alpha_beta(-player, alpha, beta, depth - 1); // 递归搜索下一层
board[i][j] = 0; // 恢复该位置为空
if (player == 1) // MAX层
{
if (score > best_score)
{
best_score = score;
}
if (best_score > alpha)
{
alpha = best_score;
}
if (beta <= alpha) // 剪枝
{
return best_score;
}
}
else // MIN层
{
if (score < best_score)
{
best_score = score;
}
if (best_score < beta)
{
beta = best_score;
}
if (beta <= alpha) // 剪枝
{
return best_score;
}
}
}
}
return best_score;
}
int find_best_move(int player) // 找到最佳落子位置
{
int best_score = player == 1 ? -9999 : 9999;
int best_move = -1;
for (int i = 0; i < BOARD_SIZE; i++)
{
for (int j = 0; j < BOARD_SIZE; j++)
{
if (board[i][j] != 0) // 如果该位置已经有棋子,跳过
{
continue;
}
board[i][j] = player; // 在该位置落子
int score = alpha_beta(-player, -9999, 9999, depth); // 使用Alpha-Beta剪枝搜索算法搜索
board[i][j] = 0; // 恢复该位置为空
if (player == 1) // MAX层
{
if (score > best_score)
{
best_score = score;
best_move = i * BOARD_SIZE + j;
}
}
else // MIN层
{
if (score < best_score)
{
best_score = score;
best_move = i * BOARD_SIZE + j;
}
}
}
}
return best_move;
}
int main()
{
// 初始化棋盘
for (int i = 0; i < BOARD_SIZE; i++)
{
for (int j = 0; j < BOARD_SIZE; j++)
{
board[i][j] = 0;
}
}
// TODO: 实现游戏逻辑
return 0;
}
```
说明:
1. evaluate函数是评估函数,用于估算当前局面的价值。该函数的实现需要根据具体情况进行调整。
2. is_win函数用于判断是否获胜。
3. alpha_beta函数是Alpha-Beta剪枝搜索算法的实现。该函数接受四个参数:player表示当前玩家,alpha和beta表示当前搜索区间,depth表示当前搜索深度。在每一层搜索时,如果达到搜索深度或者获胜,就返回当前估值。否则,在所有空位置上尝试落子,递归搜索下一层,并根据当前层是MAX层还是MIN层更新最佳分数和当前搜索区间。如果搜索区间不符合alpha-beta剪枝的要求,就进行剪枝操作。最后返回最佳分数。
4. find_best_move函数是找到最佳落子位置的函数。该函数遍历所有空位置,使用Alpha-Beta剪枝搜索算法搜索下一步的估值,并返回最佳落子位置。
5. main函数是程序的入口函数,需要实现游戏逻辑。
相关推荐
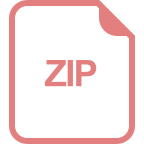
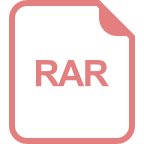
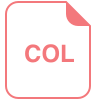
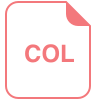

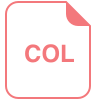










