YOLOv3的损失 用pytorch复现
时间: 2023-11-14 17:12:52 浏览: 225
YOLOv3的损失函数主要包括三个部分:物体置信度损失、类别损失和边界框损失。其中,物体置信度损失和类别损失采用交叉熵损失函数计算,边界框损失采用均方误差损失函数计算。
以下是用PyTorch复现YOLOv3的损失函数的代码:
```python
import torch
import torch.nn.functional as F
def yolo_loss(pred, target, anchors):
"""
pred: [batch_size, num_anchors*(5+num_classes), grid_size, grid_size]
target: [batch_size, max_objects, 6]
anchors: [num_anchors, 2]
"""
batch_size, num_anchors_times, grid_size, _ = pred.size()
num_anchors = len(anchors)
# 将pred转换成[batch_size, num_anchors, 5+num_classes, grid_size, grid_size]
pred = pred.view(batch_size, num_anchors, num_anchors_times//num_anchors, grid_size, grid_size).permute(0, 1, 3, 4, 2).contiguous()
# 获取预测框的中心点、宽度、高度和物体置信度
pred_xy = torch.sigmoid(pred[..., :2]) # [batch_size, num_anchors, grid_size, grid_size, 2]
pred_wh = torch.exp(pred[..., 2:4]) * anchors.view(1, num_anchors, 1, 1, 2) # [batch_size, num_anchors, grid_size, grid_size, 2]
pred_obj = torch.sigmoid(pred[..., 4:5]) # [batch_size, num_anchors, grid_size, grid_size, 1]
pred_cls = pred[..., 5:] # [batch_size, num_anchors, grid_size, grid_size, num_classes]
# 获取目标框的中心点、宽度、高度和物体置信度
target_xy = target[..., :2].unsqueeze(1) # [batch_size, 1, max_objects, 2]
target_wh = target[..., 2:4].unsqueeze(1) # [batch_size, 1, max_objects, 2]
target_obj = target[..., 4:5].unsqueeze(1) # [batch_size, 1, max_objects, 1]
target_cls = target[..., 5:].unsqueeze(1) # [batch_size, 1, max_objects, num_classes]
# 计算预测框与目标框之间的IOU
pred_min = pred_xy - pred_wh / 2
pred_max = pred_xy + pred_wh / 2
target_min = target_xy - target_wh / 2
target_max = target_xy + target_wh / 2
inter_min = torch.max(pred_min, target_min)
inter_max = torch.min(pred_max, target_max)
inter_wh = torch.clamp(inter_max - inter_min, min=0)
inter_area = inter_wh[..., 0] * inter_wh[..., 1]
pred_area = pred_wh[..., 0] * pred_wh[..., 1]
target_area = target_wh[..., 0] * target_wh[..., 1]
iou = inter_area / (pred_area + target_area - inter_area + 1e-16)
# 计算物体置信度损失
obj_mask = (iou > 0.5).float() * target_obj
noobj_mask = (iou < 0.4).float() * (1 - target_obj)
obj_loss = F.binary_cross_entropy(pred_obj, obj_mask, reduction='sum')
noobj_loss = F.binary_cross_entropy(pred_obj, noobj_mask, reduction='sum')
# 计算类别损失
cls_mask = target_obj
cls_loss = F.binary_cross_entropy_with_logits(pred_cls, cls_mask, reduction='none')
cls_loss = cls_loss * cls_mask
cls_loss = cls_loss.sum(dim=-1).sum(dim=-1).sum(dim=-1)
# 计算边界框损失
coord_mask = target_obj.unsqueeze(-1)
coord_loss = F.mse_loss(pred_xy * coord_mask, target_xy * coord_mask, reduction='sum')
coord_loss += F.mse_loss(pred_wh.sqrt() * coord_mask, target_wh.sqrt() * coord_mask, reduction='sum')
# 总损失
loss = obj_loss + noobj_loss + cls_loss + coord_loss
return loss
```
上述代码中,`pred`表示预测值,`target`表示目标值,`anchors`表示锚点框。其中,`pred`的形状为`[batch_size, num_anchors_times*(5+num_classes), grid_size, grid_size]`,`target`的形状为`[batch_size, max_objects, 6]`,`anchors`的形状为`[num_anchors, 2]`。在函数内部,首先将`pred`转换成`[batch_size, num_anchors, 5+num_classes, grid_size, grid_size]`的形状,然后获取预测框的中心点、宽度、高度和物体置信度,获取目标框的中心点、宽度、高度和物体置信度,计算预测框与目标框之间的IOU,最后根据公式计算物体置信度损失、类别损失和边界框损失,并将它们相加得到总损失。
阅读全文
相关推荐
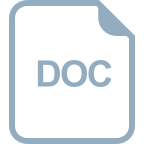
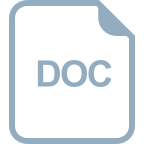
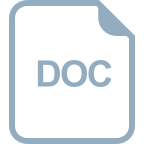

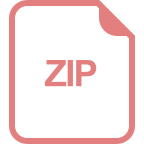
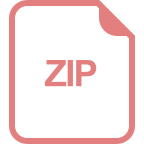
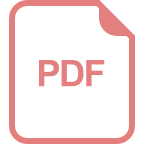
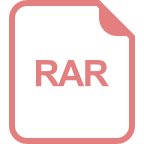
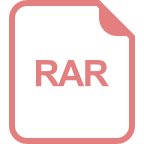
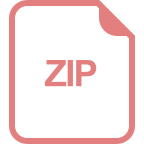
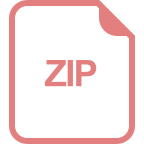
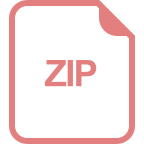
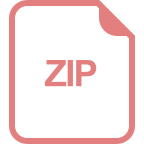
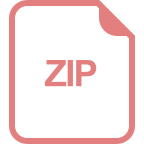
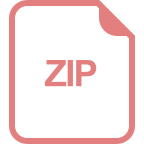
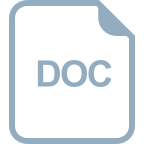
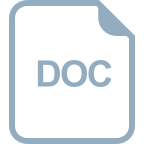

