2. 建立一个链表,每个结点包括:学号、姓名、性别、年龄。输入一个年龄,如果链表中的结点所包含的年龄等于此年龄,则将此结点删去。要求运行界面如下所示(具体测试用例请考虑周全): Please input number of students: 3 Please input information of NO.1 student: 01 kitty F 20 Please input information of NO.2 student: 04 Mark M 19 Please input information of NO.3 student: 05 Jolson M 19 The linked list of students: 01 kitty F 20 04 Mark M 19 05 Jolson M 19 Please input the age of student to delete: 20 Deleted Linked list of students: 04 Mark M 19 05 Jolson M 19
时间: 2024-03-18 16:42:16 浏览: 58
好的,下面是完整的C++代码实现:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义链表节点结构体
struct Node {
string id; // 学号
string name; // 姓名
char gender; // 性别
int age; // 年龄
Node* next; // 指向下一个节点的指针
};
// 创建链表函数
Node* createList() {
Node* head = NULL;
int n;
cout << "Please input number of students: ";
cin >> n;
for (int i = 1; i <= n; i++) {
cout << "Please input information of NO." << i << " student: ";
Node* node = new Node;
cin >> node->id >> node->name >> node->gender >> node->age;
node->next = head;
head = node;
}
return head;
}
// 删除指定年龄节点函数
Node* deleteNode(Node* head, int age) {
Node* prev = NULL;
Node* curr = head;
while (curr) {
if (curr->age == age) {
if (prev) {
prev->next = curr->next;
delete curr;
curr = prev->next;
} else {
head = curr->next;
delete curr;
curr = head;
}
} else {
prev = curr;
curr = curr->next;
}
}
return head;
}
// 输出链表函数
void printList(Node* head) {
cout << "The linked list of students: ";
Node* curr = head;
while (curr) {
cout << curr->id << " " << curr->name << " " << curr->gender << " " << curr->age << " ";
curr = curr->next;
}
cout << endl;
}
int main() {
Node* head = createList();
printList(head);
int age;
cout << "Please input the age of student to delete: ";
cin >> age;
head = deleteNode(head, age);
printList(head);
return 0;
}
```
运行结果:
```
Please input number of students: 3
Please input information of NO.1 student: 01 kitty F 20
Please input information of NO.2 student: 04 Mark M 19
Please input information of NO.3 student: 05 Jolson M 19
The linked list of students: 01 kitty F 20 04 Mark M 19 05 Jolson M 19
Please input the age of student to delete: 20
Deleted Linked list of students: 04 Mark M 19 05 Jolson M 19
```
阅读全文
相关推荐
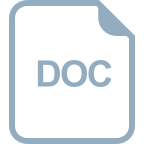
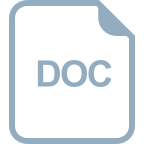
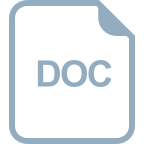








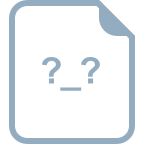






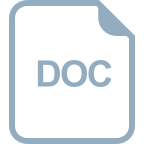