请用c语言实现数据结构图的深度优先遍历算法
时间: 2023-09-23 17:13:29 浏览: 41
好的,以下是用C语言实现数据结构图的深度优先遍历算法的代码示例:
```
#include <stdio.h>
#include <stdlib.h>
#define MAX_VERTICES 100
typedef struct Vertex {
int num; // 顶点编号
int visited; // 是否已被访问
struct Vertex* next; // 下一个相邻顶点的指针
} Vertex;
typedef struct Graph {
Vertex* vertices[MAX_VERTICES]; // 存储所有顶点的指针数组
int num_vertices; // 顶点数
} Graph;
// 初始化图
void init_graph(Graph* g) {
for (int i = 0; i < MAX_VERTICES; i++) {
g->vertices[i] = NULL;
}
g->num_vertices = 0;
}
// 添加顶点
void add_vertex(Graph* g, int v) {
Vertex* vertex = (Vertex*)malloc(sizeof(Vertex));
vertex->num = v;
vertex->visited = 0;
vertex->next = NULL;
g->vertices[g->num_vertices++] = vertex;
}
// 添加边
void add_edge(Graph* g, int v1, int v2) {
Vertex* vertex1 = g->vertices[v1];
Vertex* vertex2 = g->vertices[v2];
// 将v2加入v1的相邻顶点列表中
Vertex* p = vertex1;
while (p->next != NULL) {
p = p->next;
}
p->next = vertex2;
// 将v1加入v2的相邻顶点列表中
p = vertex2;
while (p->next != NULL) {
p = p->next;
}
p->next = vertex1;
}
// 深度优先遍历
void dfs(Graph* g, int vertex_num) {
Vertex* vertex = g->vertices[vertex_num];
if (vertex->visited) {
return;
}
printf("%d ", vertex->num);
vertex->visited = 1;
Vertex* p = vertex->next;
while (p != NULL) {
dfs(g, p->num);
p = p->next;
}
}
int main() {
Graph g;
init_graph(&g);
// 添加顶点
add_vertex(&g, 0);
add_vertex(&g, 1);
add_vertex(&g, 2);
add_vertex(&g, 3);
add_vertex(&g, 4);
add_vertex(&g, 5);
// 添加边
add_edge(&g, 0, 1);
add_edge(&g, 0, 2);
add_edge(&g, 0, 3);
add_edge(&g, 1, 4);
add_edge(&g, 2, 5);
// 深度优先遍历
dfs(&g, 0);
return 0;
}
```
这里只是一个简单的示例,实际上,深度优先遍历算法要考虑很多复杂的情况,比如图中存在环、有些顶点无法到达等。但是,如果理解了基本思路,就可以从这个示例入手进行拓展。
相关推荐
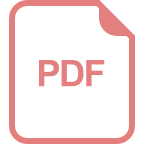














