用STM32C语言写一段驱动步进电机的代码
时间: 2024-02-19 09:59:19 浏览: 217
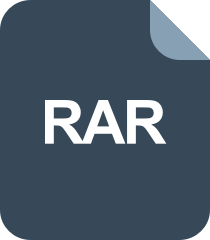
STM32c8t6启动步进电机.rar

以下是一个简单的步进电机驱动程序示例,使用STM32的HAL库和GPIO引脚控制。该示例代码使用的是4相8线步进电机,每步的角度为1.8度。
```c
#include "stm32f4xx_hal.h"
// 此处定义GPIO引脚,用于控制步进电机
#define STEP_PIN_1 GPIO_PIN_0
#define STEP_PIN_2 GPIO_PIN_1
#define STEP_PIN_3 GPIO_PIN_2
#define STEP_PIN_4 GPIO_PIN_3
#define STEP_PORT GPIOB
// 步进电机运动时的4种状态
uint8_t step_seq[4][4] = {
{1, 0, 0, 0},
{0, 1, 0, 0},
{0, 0, 1, 0},
{0, 0, 0, 1}
};
// 定义电机旋转方向
typedef enum {
CW = 0, // 顺时针
CCW = 1 // 逆时针
} direction_t;
// 定义步进电机的当前状态
uint8_t current_step = 0;
// 控制步进电机旋转
void step_motor_move(direction_t dir) {
if (dir == CW) {
current_step = (current_step + 1) % 4;
} else {
current_step = (current_step - 1 + 4) % 4;
}
HAL_GPIO_WritePin(STEP_PORT, STEP_PIN_1, step_seq[current_step][0]);
HAL_GPIO_WritePin(STEP_PORT, STEP_PIN_2, step_seq[current_step][1]);
HAL_GPIO_WritePin(STEP_PORT, STEP_PIN_3, step_seq[current_step][2]);
HAL_GPIO_WritePin(STEP_PORT, STEP_PIN_4, step_seq[current_step][3]);
}
// 主函数
int main(void) {
// 初始化GPIO引脚
GPIO_InitTypeDef GPIO_InitStruct;
__HAL_RCC_GPIOB_CLK_ENABLE();
GPIO_InitStruct.Pin = STEP_PIN_1 | STEP_PIN_2 | STEP_PIN_3 | STEP_PIN_4;
GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP;
GPIO_InitStruct.Pull = GPIO_NOPULL;
GPIO_InitStruct.Speed = GPIO_SPEED_FREQ_LOW;
HAL_GPIO_Init(STEP_PORT, &GPIO_InitStruct);
// 循环控制步进电机旋转
while (1) {
step_motor_move(CW);
HAL_Delay(10); // 延时等待电机旋转
}
}
```
这只是一个简单的示例代码,实际的步进电机驱动代码需要根据具体的电机型号和参数进行调整和优化。
阅读全文
相关推荐
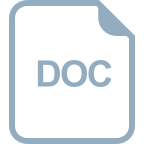
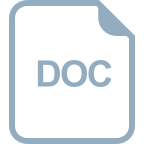










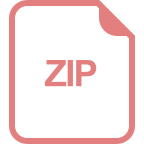
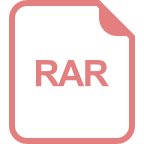



