图像坐标系转相机坐标系的代码
时间: 2023-10-02 18:04:40 浏览: 62
图像坐标系转相机坐标系的代码需要用到相机的内参矩阵以及相机的外参矩阵。假设相机内参矩阵为K,外参矩阵为[R|t],其中R为旋转矩阵,t为平移向量。设(x,y)为图像坐标系下的坐标,(X,Y,Z)为相机坐标系下的坐标,则图像坐标系转相机坐标系的代码如下:
```python
import numpy as np
def image_to_camera_coords(x, y, depth, K, R, t):
# 将图像坐标系下的坐标转化为归一化平面坐标系下的坐标
x_norm = (x - K[0, 2]) / K[0, 0]
y_norm = (y - K[1, 2]) / K[1, 1]
# 将归一化平面坐标系下的坐标转化为相机坐标系下的坐标
P_cam = np.array([x_norm, y_norm, 1.0]) * depth
# 将相机坐标系下的坐标转化为世界坐标系下的坐标
P_world = np.dot(R.T, P_cam - t)
return P_world
```
其中,x和y为图像坐标系下的坐标,depth为物体到相机的距离,K为相机的内参矩阵,R和t为相机的外参矩阵。函数的返回值为相机坐标系下的坐标。
相关问题
世界坐标系、相机坐标系、图像坐标系、像素坐标系之间的转换代码
以下是世界坐标系、相机坐标系、图像坐标系、像素坐标系之间的转换代码:
1. 世界坐标系转相机坐标系:
```
import numpy as np
def world_to_camera(world_point, R, t):
"""
世界坐标系转相机坐标系
:param world_point: 世界坐标系中的点,shape=(3,)
:param R: 相机姿态的旋转矩阵,shape=(3, 3)
:param t: 相机姿态的平移向量,shape=(3,)
:return: 相机坐标系中的点,shape=(3,)
"""
camera_point = np.dot(R, world_point) + t
return camera_point
```
2. 相机坐标系转世界坐标系:
```
import numpy as np
def camera_to_world(camera_point, R, t):
"""
相机坐标系转世界坐标系
:param camera_point: 相机坐标系中的点,shape=(3,)
:param R: 相机姿态的旋转矩阵,shape=(3, 3)
:param t: 相机姿态的平移向量,shape=(3,)
:return: 世界坐标系中的点,shape=(3,)
"""
world_point = np.dot(np.linalg.inv(R), camera_point - t)
return world_point
```
3. 相机坐标系转图像坐标系:
```
import numpy as np
def camera_to_image(camera_point, K):
"""
相机坐标系转图像坐标系
:param camera_point: 相机坐标系中的点,shape=(3,)
:param K: 相机内参矩阵,shape=(3, 3)
:return: 图像坐标系中的点,shape=(2,)
"""
image_point = np.dot(K, camera_point[:3])
image_point = image_point[:2] / image_point[2]
return image_point
```
4. 图像坐标系转像素坐标系:
```
def image_to_pixel(image_point):
"""
图像坐标系转像素坐标系
:param image_point: 图像坐标系中的点,shape=(2,)
:return: 像素坐标系中的点,shape=(2,)
"""
pixel_point = np.round(image_point).astype(int)
return pixel_point
```
5. 像素坐标系转图像坐标系:
```
def pixel_to_image(pixel_point):
"""
像素坐标系转图像坐标系
:param pixel_point: 像素坐标系中的点,shape=(2,)
:return: 图像坐标系中的点,shape=(2,)
"""
image_point = pixel_point.astype(float)
return image_point
```
世界坐标系、相机坐标系、图像坐标系、像素坐标系之间的相互转换c++代码
以下是世界坐标系、相机坐标系、图像坐标系、像素坐标系之间的相互转换的C++代码示例:
```cpp
// 定义世界坐标系中的三维点
cv::Point3f world_point(1.0, 2.0, 3.0);
// 定义相机内参矩阵
cv::Mat camera_matrix = (cv::Mat_<double>(3,3) << fx, 0, cx, 0, fy, cy, 0, 0, 1);
// 定义相机外参矩阵
cv::Mat rotation_vector, translation_vector;
cv::solvePnP(object_points, image_points, camera_matrix, cv::noArray(), rotation_vector, translation_vector);
// 将世界坐标系中的三维点转换到相机坐标系中
cv::Mat world_point_mat = (cv::Mat_<double>(3,1) << world_point.x, world_point.y, world_point.z);
cv::Mat camera_point_mat;
cv::projectPoints(world_point_mat, rotation_vector, translation_vector, camera_matrix, cv::noArray(), camera_point_mat);
// 将相机坐标系中的点转换到图像坐标系中
cv::Point2f camera_point(camera_point_mat.at<double>(0,0), camera_point_mat.at<double>(1,0));
cv::Point2f image_point;
image_point.x = camera_point.x * fx + cx;
image_point.y = camera_point.y * fy + cy;
// 将图像坐标系中的点转换到像素坐标系中
cv::Point pixel_point(cvRound(image_point.x), cvRound(image_point.y));
```
其中,`fx`、`fy`、`cx`、`cy`分别为相机内参矩阵中的元素,`object_points`和`image_points`分别为世界坐标系中的三维点和对应的图像坐标系中的二维点。
相关推荐
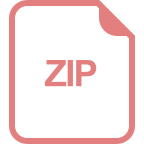
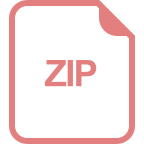












