用java写出:给一棵有n个点的有根树,点的编号为1到n,根为1。每个点的颜色是红色或者蓝色。对于树上的一个点,如果其子树中(不包括该点本身)红色点和蓝色点的数量相同,那么我们称该点是平衡的。 请你计算给定的树中有多少个点是平衡点。
时间: 2023-04-08 16:01:00 浏览: 219
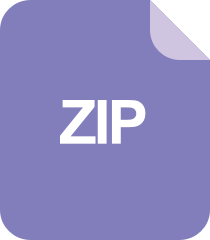
绘制树:用于可视化一般、有向或无向、有根树的学习工具。-matlab开发
可以回答这个问题。可以使用深度优先搜索(DFS)来遍历整棵树,对于每个节点,记录其子树中红色点和蓝色点的数量,然后判断是否平衡。如果平衡,则计数器加一。最后返回计数器的值即可。以下是Java代码实现:
```
import java.util.*;
public class BalancedPoint {
static int[] redCount; // 红色点的数量
static int[] blueCount; // 蓝色点的数量
static int count = 0; // 平衡点的数量
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
redCount = new int[n + 1];
blueCount = new int[n + 1];
for (int i = 1; i <= n; i++) {
int color = sc.nextInt();
if (color == 1) {
redCount[i]++;
} else {
blueCount[i]++;
}
}
List<Integer>[] graph = new List[n + 1];
for (int i = 1; i <= n; i++) {
graph[i] = new ArrayList<>();
}
for (int i = 1; i < n; i++) {
int u = sc.nextInt();
int v = sc.nextInt();
graph[u].add(v);
}
dfs(1, graph);
System.out.println(count);
}
private static void dfs(int u, List<Integer>[] graph) {
for (int v : graph[u]) {
dfs(v, graph);
redCount[u] += redCount[v];
blueCount[u] += blueCount[v];
}
if (redCount[u] == blueCount[u] && u != 1) {
count++;
}
}
}
```
阅读全文
相关推荐
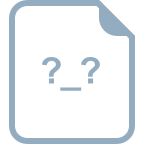
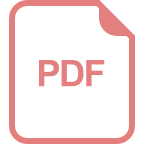









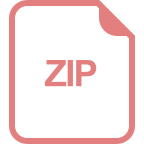
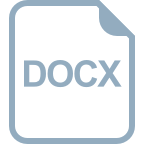
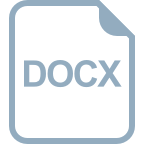
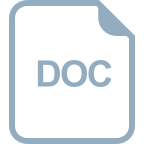
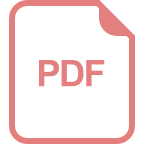