构建图的最小生成树或求最短路径。(二选一部分) (1)、用Prim法求连通图的最小生成树(邻接表) (2)、用Dijkstra算法求某一确定顶点到所有其它顶点的最短路径,请在网上寻找到这段话
时间: 2024-02-12 11:06:13 浏览: 23
好的,以下是用Prim算法求连通图的最小生成树的Python实现(使用邻接表表示图):
```python
import heapq
def prim(graph):
# 任选一个顶点作为起点
start = list(graph.keys())[0]
# 初始化距离字典、已知最短路径集合和边集合
distance = {vertex: float('inf') for vertex in graph}
distance[start] = 0
visited = set()
edges = []
# 堆优化的Prim算法
heap = [(0, start)]
while heap:
(dist, current_vertex) = heapq.heappop(heap)
# 如果当前顶点已经在已知最短路径集合中,跳过
if current_vertex in visited:
continue
visited.add(current_vertex)
# 将当前顶点与已知最短路径集合中的顶点之间的边加入边集合中
if current_vertex != start:
edges.append((distance[current_vertex], current_vertex, parent))
# 更新与当前顶点相邻的顶点的距离
for neighbor, weight in graph[current_vertex].items():
if neighbor not in visited:
if weight < distance[neighbor]:
distance[neighbor] = weight
heapq.heappush(heap, (weight, neighbor))
parent = current_vertex
# 返回边集合
return edges
```
其中,graph是邻接表表示的图。这个实现中使用了堆优化,可以有效地加速算法。
以下是用Dijkstra算法求某一确定顶点到所有其它顶点的最短路径的Python实现(使用邻接表表示图):
```python
import heapq
def dijkstra(graph, start):
# 初始化距离字典和已知最短路径集合
distance = {vertex: float('inf') for vertex in graph}
distance[start] = 0
visited = set()
# 堆优化的Dijkstra算法
heap = [(0, start)]
while heap:
(dist, current_vertex) = heapq.heappop(heap)
# 如果当前顶点已经在已知最短路径集合中,跳过
if current_vertex in visited:
continue
visited.add(current_vertex)
# 更新与当前顶点相邻的顶点的距离
for neighbor, weight in graph[current_vertex].items():
if neighbor not in visited:
new_distance = dist + weight
if new_distance < distance[neighbor]:
distance[neighbor] = new_distance
heapq.heappush(heap, (new_distance, neighbor))
# 返回距离字典
return distance
```
其中,graph是邻接表表示的图,start是起点。这个实现中使用了堆优化,可以有效地加速算法。
相关推荐
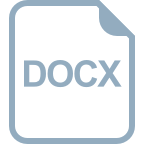
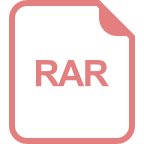
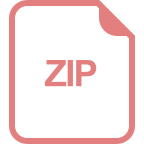














