如何在carla中设置4个NPC车辆,并将这4个NPC车辆的实时速度与玩家车辆之间的距离放在一个新的csv表里
时间: 2024-03-17 19:43:02 浏览: 33
要在CARLA中设置4个NPC车辆并将它们的实时速度和与玩家车辆之间的距离放在一个新的CSV表中,您可以执行以下步骤:
1. 在CARLA模拟器中打开一个新的场景,并将玩家车辆放置在其中。
2. 使用Python API创建4个NPC车辆并将它们放置在场景中。
3. 使用CARLA的Python API获取玩家车辆和4个NPC车辆的实时速度和位置信息。
4. 计算每个NPC车辆与玩家车辆之间的距离。
5. 将速度和距离信息存储在一个新的CSV文件中。
以下是示例代码,可以帮助您完成这项任务:
``` python
import carla
import csv
# Connect to the CARLA simulator
client = carla.Client('localhost', 2000)
client.set_timeout(10.0)
world = client.get_world()
# Create a blueprint of a vehicle
vehicle_bp = world.get_blueprint_library().find('vehicle.tesla.model3')
# Spawn the player vehicle
player_transform = carla.Transform(carla.Location(x=50, y=50, z=2), carla.Rotation(yaw=0))
player_vehicle = world.spawn_actor(vehicle_bp, player_transform)
# Spawn the NPC vehicles
npc_transforms = [
carla.Transform(carla.Location(x=100, y=50, z=2), carla.Rotation(yaw=0)),
carla.Transform(carla.Location(x=50, y=100, z=2), carla.Rotation(yaw=90)),
carla.Transform(carla.Location(x=0, y=50, z=2), carla.Rotation(yaw=180)),
carla.Transform(carla.Location(x=50, y=0, z=2), carla.Rotation(yaw=270)),
]
npc_vehicles = []
for transform in npc_transforms:
npc_vehicle = world.spawn_actor(vehicle_bp, transform)
npc_vehicles.append(npc_vehicle)
# Open a new CSV file
csv_file = open('speed_distance.csv', 'w', newline='')
csv_writer = csv.writer(csv_file)
# Loop to get the speed and distance data
while True:
# Get the player vehicle's speed and location
player_speed = player_vehicle.get_velocity().x
player_location = player_vehicle.get_location()
# Loop through each NPC vehicle
for i, npc_vehicle in enumerate(npc_vehicles):
# Get the NPC vehicle's speed and location
npc_speed = npc_vehicle.get_velocity().x
npc_location = npc_vehicle.get_location()
# Calculate the distance between the player and NPC vehicle
distance = player_location.distance(npc_location)
# Write the data to the CSV file
csv_writer.writerow([i, npc_speed, distance, player_speed])
# Wait for a short time before getting the next data point
world.wait_for_tick()
# Close the CSV file and clean up the actors
csv_file.close()
for vehicle in npc_vehicles + [player_vehicle]:
vehicle.destroy()
```
此代码可以在CARLA中设置4个NPC车辆,计算它们和玩家车之间的距离,并将数据写入新的CSV文件中。
相关推荐
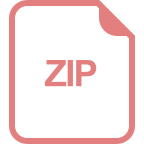
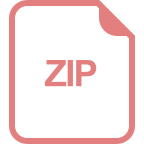














