Unity代码获取子物体的世界坐标
时间: 2024-03-09 12:50:17 浏览: 48
获取子物体的世界坐标可以使用 Transform.TransformPoint() 方法。你可以先获取子物体相对于父物体的局部坐标,然后使用该方法将其转换为世界坐标。以下是示例代码:
```csharp
// 获取子物体的 Transform 组件
Transform childTransform = transform.Find("子物体名称");
// 获取子物体的局部坐标
Vector3 localPosition = childTransform.localPosition;
// 将局部坐标转换为世界坐标
Vector3 worldPosition = childTransform.TransformPoint(localPosition);
Debug.Log("子物体的世界坐标为:" + worldPosition);
```
以上代码中,先使用 transform.Find() 方法获取子物体的 Transform 组件,然后使用 Transform.localPosition 属性获取子物体相对于父物体的局部坐标。最后使用 Transform.TransformPoint() 方法将局部坐标转换为世界坐标。
相关问题
unity 获取移动物体的坐标
在 Unity 中,你可以使用 transform 组件来获取移动物体的坐标。transform 组件包含了物体的位置、旋转和缩放信息。
要获取移动物体的位置坐标,你可以使用 transform.position 属性。下面是一个示例代码:
```csharp
using UnityEngine;
public class GetObjectPosition : MonoBehaviour
{
private void Start()
{
// 获取当前物体的位置坐标
Vector3 objectPosition = transform.position;
Debug.Log("Object Position: " + objectPosition);
}
}
```
在上述示例中,我们在 Start 方法中获取了当前物体的位置坐标,并使用 Debug.Log 输出到控制台。
希望对你有所帮助!如有更多问题,请随时提问。
在unity中获取世界坐标
在Unity中,可以通过使用Transform组件的position属性获取物体的世界坐标。例如:
```
Transform transform;
Vector3 worldPos;
void Start () {
transform = GetComponent<Transform>();
worldPos = transform.position;
}
```
这段代码会在物体启动时获取该物体的Transform组件,并将它的世界坐标存储在worldPos变量中。
相关推荐
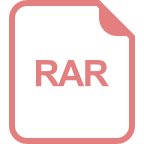
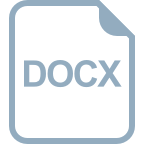












