unity控制物体局部坐标的代码
时间: 2024-09-24 14:21:49 浏览: 31
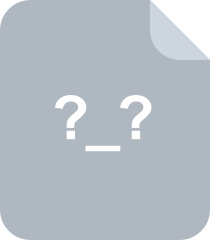
Unity 3D 观察物体 旋转查看物体 世界坐标与局部坐标旋转转换 旋转物体问题

在Unity中,如果你想控制游戏物体的局部坐标(Local Coordinates),你可以使用Transform组件的一些方法。例如,Transform类有`Translate()`、`Rotate()`和`Scale()`方法,分别用于移动、旋转和缩放游戏对象。
如果你想只改变局部坐标,不应用到世界空间,可以这样做:
```csharp
// 获取当前物体的Transform组件
Transform myObject = GetComponent<Transform>();
// 移动物体的局部位置
myObject.localPosition = new Vector3(x, y, z); // x, y, z是你想要的新位置
// 旋转物体的局部旋转
Quaternion rotation = Quaternion.Euler(xRotation, yRotation, zRotation);
myObject.localRotation = rotation;
// 缩放物体的局部大小
myObject.localScale = new Vector3(scaleX, scaleY, scaleZ); // scaleX, scaleY, scaleZ分别是新的缩放比例
```
在这个例子中,`x`, `y`, `z`, `xRotation`, `yRotation`, `zRotation`, `scaleX`, `scaleY`, `scaleZ`都是你要设置的具体数值。
阅读全文
相关推荐
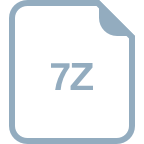
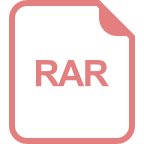















