编写一个程序,递归调用被继承的基类成员函数,实现求素数与最大公约数的功能
时间: 2024-02-16 11:02:58 浏览: 108
好的,我可以帮你编写这个程序。以下是一个示例代码,你可以参考一下:
```c++
#include <iostream>
using namespace std;
class Math {
public:
virtual bool isPrime(int n) {
if (n < 2) return false;
for (int i = 2; i <= n / 2; i++) {
if (n % i == 0) return false;
}
return true;
}
virtual int gcd(int a, int b) {
if (b == 0) return a;
return gcd(b, a % b);
}
};
class NewMath : public Math {
public:
virtual bool isPrime(int n) {
if (!Math::isPrime(n)) return false;
if (n == 2 || n == 3) return true;
if (n % 6 != 1 && n % 6 != 5) return false;
for (int i = 5; i * i <= n; i += 6) {
if (n % i == 0 || n % (i + 2) == 0) return false;
}
return true;
}
};
int main() {
NewMath nm;
cout << "Is 7 a prime number? " << nm.isPrime(7) << endl;
cout << "Is 12 a prime number? " << nm.isPrime(12) << endl;
cout << "GCD of 12 and 18: " << nm.gcd(12, 18) << endl;
cout << "GCD of 24 and 36: " << nm.gcd(24, 36) << endl;
return 0;
}
```
这个程序定义了一个名为`Math`的基类,其中包含了两个虚函数,分别用于判断一个整数是否为素数和求两个整数的最大公约数。然后,定义了一个名为`NewMath`的类,它继承了`Math`类,并覆盖了`isPrime`函数。
在`NewMath`的`isPrime`函数中,我们首先调用了基类的`isPrime`函数,如果这个函数返回`false`,则说明这个数字一定不是素数,直接返回`false`即可。接着,我们使用了更加高效的判断素数的算法,即“6n+1”和“6n-1”算法。最后,在`main`函数中,我们创建了一个`NewMath`对象,并使用它来检查一些数字是否为素数,以及计算一些数字的最大公约数。
希望这个程序能够帮到你!
阅读全文
相关推荐
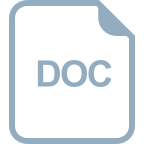
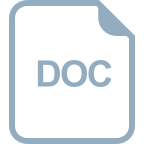
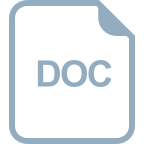














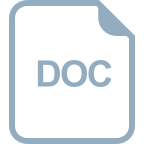