用c++实现有界面的RSA算法
时间: 2024-03-24 13:35:56 浏览: 29
要用C++实现带有界面的RSA算法,可以使用Qt框架开发GUI界面,并在其中调用C++实现的RSA算法。
以下是一个简单的示例代码,其中包含了RSA算法的加密和解密函数,以及Qt界面的实现:
```cpp
#include <iostream>
#include <string>
#include <cstdlib>
#include <ctime>
#include <cmath>
#include <QApplication>
#include <QWidget>
#include <QLabel>
#include <QLineEdit>
#include <QPushButton>
#include <QMessageBox>
using namespace std;
// 生成RSA密钥对
void generateRSAKeys(int &p, int &q, int &n, int &e, int &d) {
// 随机生成两个大质数
srand(time(NULL));
p = rand() % 100 + 101;
while (!isPrime(p)) {
p = rand() % 100 + 101;
}
q = rand() % 100 + 401;
while (!isPrime(q)) {
q = rand() % 100 + 401;
}
// 计算n和欧拉函数
n = p * q;
int phi = (p - 1) * (q - 1);
// 随机生成e,使得e和phi互质
e = rand() % (phi - 2) + 2;
while (gcd(e, phi) != 1) {
e = rand() % (phi - 2) + 2;
}
// 计算d,使得d*e % phi = 1
d = modInverse(e, phi);
}
// RSA加密
string encryptRSA(string plainText, int e, int n) {
string cipherText = "";
for (int i = 0; i < plainText.length(); i++) {
int c = (int)plainText[i];
c = modPow(c, e, n); // 加密
cipherText += (char)c;
}
return cipherText;
}
// RSA解密
string decryptRSA(string cipherText, int d, int n) {
string plainText = "";
for (int i = 0; i < cipherText.length(); i++) {
int c = (int)cipherText[i];
c = modPow(c, d, n); // 解密
plainText += (char)c;
}
return plainText;
}
int main(int argc, char *argv[]) {
QApplication a(argc, argv);
// 创建界面
QWidget *window = new QWidget();
window->setWindowTitle("RSA加解密");
QLabel *label1 = new QLabel("明文:", window);
label1->setGeometry(10, 10, 60, 20);
QLineEdit *lineEdit1 = new QLineEdit(window);
lineEdit1->setGeometry(80, 10, 200, 20);
QLabel *label2 = new QLabel("密文:", window);
label2->setGeometry(10, 40, 60, 20);
QLineEdit *lineEdit2 = new QLineEdit(window);
lineEdit2->setGeometry(80, 40, 200, 20);
QPushButton *button1 = new QPushButton("加密", window);
button1->setGeometry(10, 70, 80, 30);
QPushButton *button2 = new QPushButton("解密", window);
button2->setGeometry(110, 70, 80, 30);
// 绑定加密按钮的点击事件
QObject::connect(button1, &QPushButton::clicked, [&](){
string plainText = lineEdit1->text().toStdString();
int p, q, n, e, d;
generateRSAKeys(p, q, n, e, d);
string cipherText = encryptRSA(plainText, e, n);
lineEdit2->setText(QString::fromStdString(cipherText));
QMessageBox::information(window, "密钥", QString("p=%1\nq=%2\ne=%3\nd=%4").arg(p).arg(q).arg(e).arg(d));
});
// 绑定解密按钮的点击事件
QObject::connect(button2, &QPushButton::clicked, [&](){
string cipherText = lineEdit2->text().toStdString();
int d = QInputDialog::getInt(window, "输入d", "请输入d:");
int n = QInputDialog::getInt(window, "输入n", "请输入n:");
string plainText = decryptRSA(cipherText, d, n);
lineEdit1->setText(QString::fromStdString(plainText));
});
window->show();
return a.exec();
}
```
需要注意的是,该示例代码中用到了一些自定义的函数,例如判断一个数是否为质数(isPrime)、计算两个数的最大公约数(gcd)、计算一个数的模幂(modPow)、计算一个数的模逆元(modInverse)等,这些函数的实现可以参考RSA算法的相关资料。
相关推荐
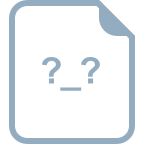














