stm32 红外循迹小车代码
时间: 2023-07-09 22:23:05 浏览: 100
以下是一个简单的红外循迹小车代码,使用 STM32F103C8T6 开发板和四路红外线传感器模块,需要自行调整引脚和代码。
```c
#include "stm32f10x.h"
// 定义红外传感器引脚
#define IR1 GPIO_Pin_0
#define IR2 GPIO_Pin_1
#define IR3 GPIO_Pin_2
#define IR4 GPIO_Pin_3
// 定义电机引脚
#define IN1 GPIO_Pin_4
#define IN2 GPIO_Pin_5
#define IN3 GPIO_Pin_6
#define IN4 GPIO_Pin_7
// 定义小车状态
#define FORWARD 0
#define LEFT 1
#define RIGHT 2
#define STOP 3
// 初始化GPIO
void GPIO_Configuration(void)
{
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_GPIOB | RCC_APB2Periph_GPIOC, ENABLE);
// 红外传感器引脚配置为输入模式
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_InitStructure.GPIO_Pin = IR1 | IR2 | IR3 | IR4;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 电机引脚配置为输出模式
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Pin = IN1 | IN2 | IN3 | IN4;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
// 读取红外传感器状态
uint8_t Read_IR(void)
{
uint8_t IR_State = 0;
if(GPIO_ReadInputDataBit(GPIOA, IR1) == RESET)
IR_State |= 0x01;
if(GPIO_ReadInputDataBit(GPIOA, IR2) == RESET)
IR_State |= 0x02;
if(GPIO_ReadInputDataBit(GPIOA, IR3) == RESET)
IR_State |= 0x04;
if(GPIO_ReadInputDataBit(GPIOA, IR4) == RESET)
IR_State |= 0x08;
return IR_State;
}
// 控制小车运动
void Car_Run(uint8_t mode)
{
switch(mode) {
case FORWARD:
GPIO_SetBits(GPIOB, IN1);
GPIO_ResetBits(GPIOB, IN2);
GPIO_SetBits(GPIOB, IN3);
GPIO_ResetBits(GPIOB, IN4);
break;
case LEFT:
GPIO_ResetBits(GPIOB, IN1);
GPIO_ResetBits(GPIOB, IN2);
GPIO_SetBits(GPIOB, IN3);
GPIO_ResetBits(GPIOB, IN4);
break;
case RIGHT:
GPIO_SetBits(GPIOB, IN1);
GPIO_ResetBits(GPIOB, IN2);
GPIO_ResetBits(GPIOB, IN3);
GPIO_ResetBits(GPIOB, IN4);
break;
case STOP:
GPIO_ResetBits(GPIOB, IN1);
GPIO_ResetBits(GPIOB, IN2);
GPIO_ResetBits(GPIOB, IN3);
GPIO_ResetBits(GPIOB, IN4);
break;
}
}
int main(void)
{
GPIO_Configuration();
while(1) {
uint8_t IR_State = Read_IR();
switch(IR_State) {
case 0x01:
Car_Run(LEFT);
break;
case 0x02:
Car_Run(FORWARD);
break;
case 0x04:
Car_Run(RIGHT);
break;
case 0x08:
Car_Run(STOP);
break;
default:
break;
}
}
}
```
希望这个代码对你有帮助。
阅读全文
相关推荐
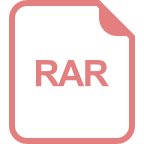
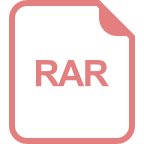



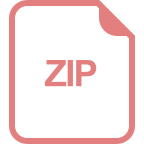
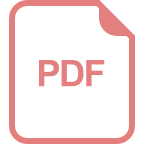








